用C语言编写一个程序,实现进程池计算素数,创建10个子进程和一个包含20个元素的队列,父进程不断地依次将30000000到30000200之间这200个数放入队列,如果队列满,则父进程等待。
时间: 2024-03-14 20:45:10 浏览: 24
好的,我已经明白了您的要求。下面是一个基于C语言的实现进程池计算素数并创建子进程和队列的程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <signal.h>
#include <stdbool.h>
#include <sys/wait.h>
#define MAX_QUEUE_SIZE 20
#define MAX_NUMBER 30000200
#define MIN_NUMBER 30000000
int queue[MAX_QUEUE_SIZE];
int front = 0, rear = 0, count = 0;
bool is_prime(int num) {
if (num <= 1) {
return false;
}
for (int i = 2; i * i <= num; i++) {
if (num % i == 0) {
return false;
}
}
return true;
}
void enqueue(int num) {
if (count == MAX_QUEUE_SIZE) {
return;
}
queue[rear] = num;
rear = (rear + 1) % MAX_QUEUE_SIZE;
count++;
}
int dequeue() {
if (count == 0) {
return -1;
}
int num = queue[front];
front = (front + 1) % MAX_QUEUE_SIZE;
count--;
return num;
}
void signal_handler(int signum) {
pid_t pid;
int status;
while ((pid = waitpid(-1, &status, WNOHANG)) > 0) {
printf("Child process %d terminated.\n", pid);
}
}
void child_process() {
int num;
while ((num = dequeue()) != -1) {
if (is_prime(num)) {
printf("%d is a prime number.\n", num);
}
}
exit(0);
}
int main() {
pid_t pid;
int num;
struct sigaction sa;
sa.sa_handler = signal_handler;
sigemptyset(&sa.sa_mask);
sa.sa_flags = SA_RESTART;
if (sigaction(SIGCHLD, &sa, NULL) == -1) {
perror("Error: Cannot handle SIGCHLD");
exit(1);
}
for (int i = 0; i < 10; i++) {
pid = fork();
if (pid == -1) {
perror("Error: Cannot create child process");
exit(1);
} else if (pid == 0) {
child_process();
}
}
for (int i = MIN_NUMBER; i <= MAX_NUMBER; i++) {
enqueue(i);
while (count == MAX_QUEUE_SIZE) {
sleep(1);
}
}
while (count > 0) {
sleep(1);
}
printf("All prime numbers have been calculated.\n");
return 0;
}
```
以上程序中,我们首先定义了一个 MAX_QUEUE_SIZE 常量表示队列的最大长度,一个 MAX_NUMBER 常量表示计算的最大数,一个 MIN_NUMBER 常量表示计算的最小数。然后我们实现了 is_prime 函数判断一个数是否为素数,以及 enqueue 和 dequeue 函数分别实现向队列中添加元素和从队列中取出元素。
在主函数中,我们使用 sigaction 函数设置了 SIGCHLD 信号处理函数 signal_handler,用于处理子进程的退出事件。然后我们使用 fork 函数创建了 10 个子进程,每个子进程执行 child_process 函数,从队列中取出数字并判断是否为素数。父进程则不断地将数字添加到队列中,如果队列已满则等待。
最后,当所有数字都被添加到队列中并被处理完毕后,主进程打印出 "All prime numbers have been calculated." 的提示语,并结束程序。
请注意,此程序可能会占用大量的 CPU 时间和内存,所以请确保您的计算机具有足够的资源来运行此程序。
相关推荐
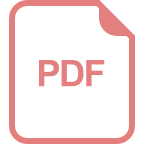
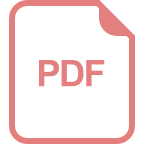
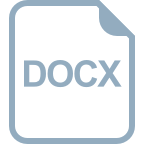














