启用六个线程,执行500个任务,并实时记录已经完成成员数 给出C++代码
时间: 2024-01-07 10:05:47 浏览: 149
以下是一个简单的C++代码示例,用于启用六个线程来执行500个任务,并实时记录已经完成任务的数量。该代码使用了C++11中的std::thread和std::atomic,以确保线程安全。
```
#include <iostream>
#include <thread>
#include <atomic>
std::atomic<int> completed_tasks(0);
void task() {
// 执行任务的代码
// ...
// 增加已完成任务数
completed_tasks++;
}
int main() {
const int num_threads = 6;
const int num_tasks = 500;
// 创建线程
std::thread threads[num_threads];
for (int i = 0; i < num_threads; i++) {
threads[i] = std::thread([](){
while (completed_tasks < num_tasks) {
task();
}
});
}
// 实时记录已完成任务数
while (completed_tasks < num_tasks) {
std::cout << "已完成任务数:" << completed_tasks << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(1));
}
// 等待线程完成
for (int i = 0; i < num_threads; i++) {
threads[i].join();
}
std::cout << "所有任务已完成!" << std::endl;
return 0;
}
```
在上面的代码中,我们首先定义了一个std::atomic<int>类型的变量completed_tasks,用于记录已完成任务数。然后,我们创建了六个线程,并将每个线程指派为一个无限循环,直到所有任务都已完成。每个线程都会调用任务函数task(),并在完成任务后将completed_tasks加1。在主线程中,我们使用一个while循环来实时记录已完成任务数,并在任务全部完成后等待所有线程结束。
阅读全文
相关推荐





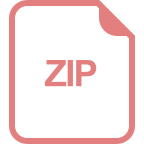
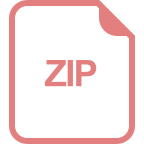
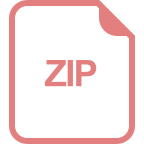
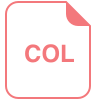
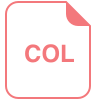





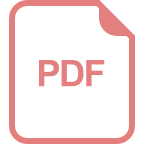