c++链表
时间: 2023-06-28 19:08:53 浏览: 43
链表是一种常见的数据结构,C++也提供了相关的链表类。下面是一个简单的单向链表的实现示例:
```c++
#include <iostream>
using namespace std;
// 链表节点
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 链表类
class LinkedList {
public:
LinkedList() {
head = NULL;
size = 0;
}
// 在链表尾部添加一个节点
void add(int val) {
ListNode* node = new ListNode(val);
if (head == NULL) {
head = node;
} else {
ListNode* cur = head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = node;
}
size++;
}
// 删除指定值的节点
void remove(int val) {
if (head == NULL) {
return;
}
if (head->val == val) {
ListNode* temp = head;
head = head->next;
delete temp;
size--;
return;
}
ListNode* cur = head;
while (cur->next != NULL) {
if (cur->next->val == val) {
ListNode* temp = cur->next;
cur->next = cur->next->next;
delete temp;
size--;
return;
}
cur = cur->next;
}
}
// 打印整个链表
void print() {
ListNode* cur = head;
while (cur != NULL) {
cout << cur->val << " ";
cur = cur->next;
}
cout << endl;
}
// 获取链表的长度
int getSize() {
return size;
}
private:
ListNode* head;
int size;
};
int main() {
LinkedList list;
list.add(1);
list.add(2);
list.add(3);
list.add(4);
list.print(); // 输出:1 2 3 4
list.remove(3);
list.print(); // 输出:1 2 4
cout << "链表长度:" << list.getSize() << endl; // 输出:链表长度:3
return 0;
}
```
这个链表类实现了在链表尾部添加一个节点、删除指定值的节点、打印整个链表、获取链表的长度等方法。你可以根据自己的需求进行修改和扩展。
相关推荐













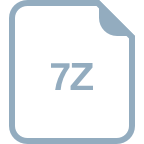