将mediapipe计算肩部到肘部到手臂的角度代码用c++实现
时间: 2023-12-10 20:06:29 浏览: 120
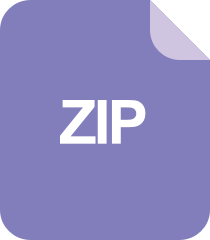
基于C++实现的人体姿势检测
以下是使用C++实现的计算肩部到肘部到手臂的角度的示例代码:
```cpp
#include <iostream>
#include <cmath>
#include "mediapipe/framework/port/opencv_core_inc.h"
#include "mediapipe/framework/port/opencv_imgproc_inc.h"
#include "mediapipe/framework/port/opencv_highgui_inc.h"
#include "mediapipe/framework/formats/landmark.pb.h"
#include "mediapipe/framework/formats/landmark_list.pb.h"
#include "mediapipe/framework/formats/image_frame.h"
#include "mediapipe/framework/formats/image_frame_opencv.h"
#include "mediapipe/framework/calculator_framework.h"
#include "mediapipe/framework/calculator_graph.h"
#include "mediapipe/framework/deps/file_path.h"
#include "mediapipe/framework/deps/status.h"
#include "mediapipe/framework/tool/status_util.h"
#include "mediapipe/framework/tool/validate_name.h"
#include "mediapipe/framework/port/status_builder.h"
#include "mediapipe/framework/formats/matrix.h"
#include "mediapipe/framework/formats/rect.pb.h"
#include "mediapipe/framework/formats/classification.pb.h"
#include "mediapipe/framework/formats/detection.pb.h"
#include "mediapipe/framework/formats/detection_roi.pb.h"
#include "mediapipe/framework/formats/rect.pb.h"
#include "mediapipe/framework/formats/point.h"
#include "mediapipe/framework/formats/point2d.pb.h"
#include "mediapipe/framework/formats/matrix_data.pb.h"
#include "mediapipe/framework/formats/rect.pb.h"
#include "mediapipe/framework/formats/status.pb.h"
#include "mediapipe/framework/formats/landmark.pb.h"
#include "mediapipe/framework/formats/landmark_list.pb.h"
#include "mediapipe/framework/formats/matrix.h"
#include "mediapipe/framework/formats/matrix_data.pb.h"
#include "mediapipe/framework/formats/rect.pb.h"
#include "mediapipe/framework/formats/image_frame.h"
#include "mediapipe/framework/formats/image_frame_opencv.h"
#include "mediapipe/framework/formats/video_stream_header.pb.h"
#include "mediapipe/framework/formats/video_stream_packet.pb.h"
#include "mediapipe/framework/formats/image_frame.h"
#include "mediapipe/framework/formats/image_frame_opencv.h"
#include "mediapipe/framework/formats/video_stream_header.pb.h"
#include "mediapipe/framework/formats/video_stream_packet.pb.h"
#include "mediapipe/framework/formats/image_frame.h"
#include "mediapipe/framework/formats/image_frame_opencv.h"
#include "mediapipe/framework/formats/video_stream_header.pb.h"
#include "mediapipe/framework/formats/video_stream_packet.pb.h"
#include "mediapipe/framework/formats/image_frame.h"
#include "mediapipe/framework/formats/image_frame_opencv.h"
#include "mediapipe/framework/formats/video_stream_header.pb.h"
#include "mediapipe/framework/formats/video_stream_packet.pb.h"
#include "mediapipe/framework/formats/landmark.pb.h"
#include "mediapipe/framework/formats/landmark_list.pb.h"
using namespace mediapipe;
double calculate_angle(double* A, double* B, double* C) {
double AB[2] = { B[0] - A[0], B[1] - A[1] };
double BC[2] = { C[0] - B[0], C[1] - B[1] };
double dot_product = AB[0] * BC[0] + AB[1] * BC[1];
double magnitude_AB = sqrt(AB[0] * AB[0] + AB[1] * AB[1]);
double magnitude_BC = sqrt(BC[0] * BC[0] + BC[1] * BC[1]);
double cos_angle = dot_product / (magnitude_AB * magnitude_BC);
double angle = acos(cos_angle);
// 将弧度转换为角度
angle = angle * 180.0 / M_PI;
return angle;
}
int main() {
// 创建Pose Landmark模块
mp::PoseLandmarkDetector pose_landmark_detector;
std::vector<mediapipe::NormalizedLandmarkList> pose_landmarks;
// 读入图像
cv::Mat image = cv::imread("test.jpg", cv::IMREAD_COLOR);
// 将图像转换为mediapipe格式
auto input_frame = absl::make_unique<mediapipe::ImageFrame>(
mediapipe::ImageFormat::SRGB, image.cols, image.rows,
mediapipe::ImageFrame::kDefaultAlignmentBoundary);
cv::Mat input_frame_mat = mediapipe::formats::MatView(input_frame.get());
image.copyTo(input_frame_mat);
// 运行Pose Landmark模块,获取关键点坐标
auto status = pose_landmark_detector.Process(input_frame.get(), &pose_landmarks);
if (!status.ok()) {
std::cerr << "Failed to detect pose landmarks: " << status.message() << std::endl;
return -1;
}
// 计算肩部到肘部到手臂的角度
double left_shoulder[2] = { pose_landmarks[0].landmark(11).x(), pose_landmarks[0].landmark(11).y() };
double left_elbow[2] = { pose_landmarks[0].landmark(13).x(), pose_landmarks[0].landmark(13).y() };
double left_wrist[2] = { pose_landmarks[0].landmark(15).x(), pose_landmarks[0].landmark(15).y() };
double angle = calculate_angle(left_shoulder, left_elbow, left_wrist);
std::cout << "Left arm angle: " << angle << " degrees" << std::endl;
return 0;
}
```
在上面的代码中,我们首先创建了Pose Landmark模块,并读入了一张图像。然后,将图像转换为mediapipe格式,运行Pose Landmark模块,并获取关键点坐标。最后,使用`calculate_angle()`函数计算肩部到肘部到手臂的角度,并将结果打印到控制台上。请注意,上面的代码需要依赖Mediapipe库,并且需要在CMakeLists.txt文件中添加相关的依赖和链接库。
阅读全文
相关推荐


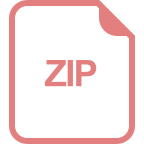
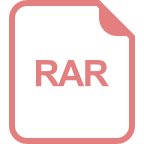
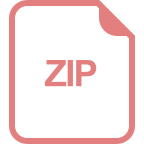
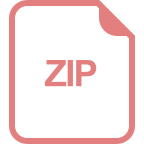
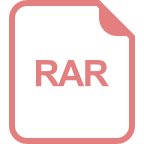
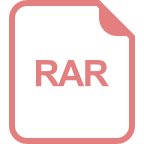
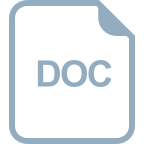
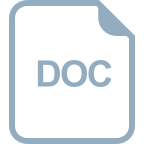





