c语言的完整二叉树创建代码
时间: 2023-10-04 20:09:49 浏览: 70
下面是C语言的完整二叉树创建代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *left;
struct node *right;
} Node;
Node* createNode(int data) {
Node *newNode = (Node*) malloc(sizeof(Node));
newNode->data = data;
newNode->left = NULL;
newNode->right = NULL;
return newNode;
}
Node* insert(Node *root, int data, int index, int n) {
if (index <= n) {
if (root == NULL) {
root = createNode(data);
} else {
root->left = insert(root->left, data, 2 * index, n);
root->right = insert(root->right, data, 2 * index + 1, n);
}
}
return root;
}
void preOrder(Node *root) {
if (root != NULL) {
printf("%d ", root->data);
preOrder(root->left);
preOrder(root->right);
}
}
int main() {
Node *root = NULL;
int n, data;
printf("Enter the number of nodes: ");
scanf("%d", &n);
printf("Enter the data for each node:\n");
for (int i = 1; i <= n; i++) {
scanf("%d", &data);
root = insert(root, data, 1, n);
}
printf("The pre-order traversal of the tree is:\n");
preOrder(root);
return 0;
}
```
在该程序中,我们使用了递归来创建完整二叉树。我们首先定义了一个节点结构体,其中包含了节点的值以及左右子节点的指针。然后我们定义了一个函数 `createNode` 用于创建新的节点。接下来,我们定义了 `insert` 函数,该函数会将新节点插入到完整二叉树中。最后,我们定义了 `preOrder` 函数,用于先序遍历该完整二叉树。
在 `main` 函数中,我们首先从用户输入中读取节点数量 `n`,然后逐个读取每个节点的值,并将其插入到完整二叉树中。最后,我们使用 `preOrder` 函数遍历该完整二叉树,并输出其先序遍历结果。
阅读全文
相关推荐
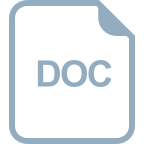
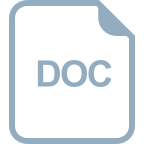
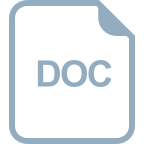
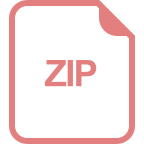









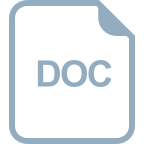
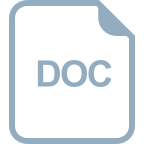
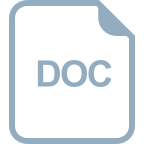
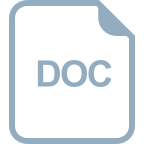
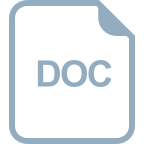
