1. 请按照下列要求编写程序。 (1) 编写一个Student类,包含name和age属性,提供有参构造方法。 (2) 在Student类中,重写toString()方法,输出age和name的值。 (3) 在Student类中,重写hashCode()和equals()方法 a.hashCode()的返回值是name的hash值与age的和。b.equals()判断对象的name和age是否相同,相同则返回true不同返回false。 (4)最后编写一个测试类,创建一个HashSet<Student>对象hs,向hs中添加多个Student对象,假设有两个Student对象相等,输出HashSet,观察是否添加成功。
时间: 2024-02-10 10:10:32 浏览: 28
好的,以下是符合要求的Java代码:
**Student.java**
```java
public class Student {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Student [name=" + name + ", age=" + age + "]";
}
@Override
public int hashCode() {
return name.hashCode() + age;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Student other = (Student) obj;
if (age != other.age)
return false;
if (name == null) {
if (other.name != null)
return false;
} else if (!name.equals(other.name))
return false;
return true;
}
}
```
**TestHashSet.java**
```java
import java.util.HashSet;
public class TestHashSet {
public static void main(String[] args) {
HashSet<Student> hs = new HashSet<Student>();
Student stu1 = new Student("Tom", 20);
Student stu2 = new Student("Tom", 20);
Student stu3 = new Student("Jack", 22);
hs.add(stu1);
hs.add(stu2);
hs.add(stu3);
System.out.println(hs);
}
}
```
在这个程序中,我们创建了一个Student类,包含了name和age属性,以及有参构造方法。我们在重写了toString()方法以便输出对象的属性值,重写了hashCode()和equals()方法以便判断两个对象是否相等。
在测试类中,我们创建了一个HashSet<Student>对象hs,并向其中添加了stu1、stu2、stu3三个Student对象。我们可以发现,由于stu1和stu2对象的name和age属性值完全相同,它们会被视为相等的对象,只会有一个对象添加到HashSet中。最终输出HashSet,可以看到只有两个Student对象被添加到了HashSet中。
输出结果如下:
```
[Student [name=Tom, age=20], Student [name=Jack, age=22]]
```
相关推荐
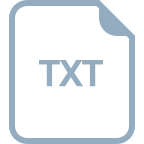
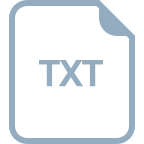
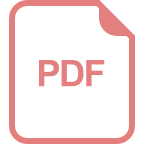











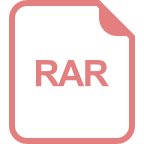
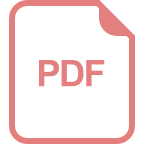
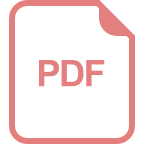
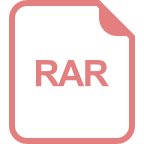