汽车收费 car的收费公式为: 载客数*8+重量*2 truck的收费公式为:重量*5 bus的收费公式为: 载客数*3 输入格式:每个测试用例占一行,每行给出汽车的基本信息,每一个为当前汽车的类型1为car,2为truck,3为bus。接下来为它的编号,接下来car是载客数和重量,truck给出重量,bus给出载客数。最后一行为0,表示输入的结束。 输出各车的编号和收费
时间: 2023-05-02 17:05:18 浏览: 89
题目要求输入每个车的载客数和重量,并按照公式计算收费,输出每个车的基本信息,包括编号、类型、载客数、重量和收费。车辆类型分为1表示car,2表示truck,3表示bus。最后一行输出0表示输入结束。
例如,输入一行: "2 100",表示编号为2的truck,载客数为0,重量为100,按照公式重量*5计算收费,输出为:"2 truck 0 100 500"。
相关问题
汽车收费\ncar的收费公式为: 载客数*8+重量*2\n\ntruck的收费公式为:重量*5\n\nbus的收费公式为: 载客数*3\n\n输入格式:每个测试用例占一行,每行给出汽车的基本信息,每一个为当前汽车的
根据题目,汽车的收费公式为:载客数*8+重量*2,卡车的收费公式为:重量*5,公共汽车的收费公式为:载客数*3。每个测试用例占一行,每行给出一个基本信息,每个基本信息为当前汽车的载客数或重量(对于每种车型分别输入,载客数或重量为0也要输入),以及车的类型(car、truck或bus)。
现在要开发一个系统,管理对多种汽车的收费工作。 给出下面的一个基类框架 class Vehicle { protected: string NO;//编号 public: virtual void display()=0;//输出应收费用 } 以Vehicle为基类,构建出Car、Truck和Bus三个派生类。 Car的收费公式为: 载客数*8+重量*2; Truck的收费公式为:重量*5; Bus的收费公式为: 载客数*3; 生成上述类并编写主函数,要求主函数中有一个基类Vehicle指针数组,数组元素不超过10个。 Vehicle *pv[10]; 主函数根据输入的信息,相应建立Car、Truck和Bus类对象,对于Car给出载客数和重量,Truck给出重量,Bus给出载客数。假设载客数和重量均为整数。提示:应用虚函数实现多态。
下面是代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
// 车辆基类
class Vehicle {
protected:
string NO; // 编号
public:
virtual void display() = 0; // 输出应收费用
};
// 小汽车类
class Car : public Vehicle {
private:
int passengerNum; // 载客数
int weight; // 重量
public:
Car(string no, int pn, int w) : passengerNum(pn), weight(w) {
NO = no;
}
void display() {
cout << "车牌号:" << NO << ",收费:" << passengerNum * 8 + weight * 2 << "元" << endl;
}
};
// 卡车类
class Truck : public Vehicle {
private:
int weight; // 重量
public:
Truck(string no, int w) : weight(w) {
NO = no;
}
void display() {
cout << "车牌号:" << NO << ",收费:" << weight * 5 << "元" << endl;
}
};
// 巴士类
class Bus : public Vehicle {
private:
int passengerNum; // 载客数
public:
Bus(string no, int pn) : passengerNum(pn) {
NO = no;
}
void display() {
cout << "车牌号:" << NO << ",收费:" << passengerNum * 3 << "元" << endl;
}
};
int main() {
int n = 0; // 车辆数量
Vehicle* pv[10]; // 指向Vehicle对象的指针数组,最多10个元素
char type; // 车辆类型
string no; // 车牌号
int pn, w; // 载客数和重量
while (true) {
cout << "请输入车辆类型(C表示小汽车,T表示卡车,B表示巴士),按0结束:" << endl;
cin >> type;
if (type == '0') break;
cout << "请输入车牌号:" << endl;
cin >> no;
switch (type) {
case 'C':
cout << "请输入载客数和重量:" << endl;
cin >> pn >> w;
pv[n++] = new Car(no, pn, w);
break;
case 'T':
cout << "请输入重量:" << endl;
cin >> w;
pv[n++] = new Truck(no, w);
break;
case 'B':
cout << "请输入载客数:" << endl;
cin >> pn;
pv[n++] = new Bus(no, pn);
break;
default:
cout << "输入错误,请重新输入!" << endl;
break;
}
if (n == 10) break; // 最多只能输入10辆车
}
cout << "收费情况如下:" << endl;
for (int i = 0; i < n; i++) {
pv[i]->display();
}
for (int i = 0; i < n; i++) {
delete pv[i]; // 释放动态分配的内存
}
return 0;
}
```
运行程序,输入车辆信息后,将输出每辆车的收费情况。注意,如果输入的车辆数量超过10辆,则只会处理前10辆车。
阅读全文
相关推荐
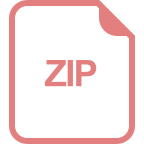
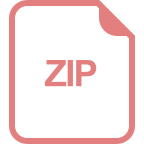
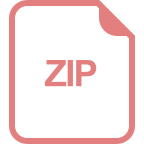














