帮我看看我修改后的代码有什么问题:#include<iostream> #include<string> using namespace std; class Vehicle { protected: string no; //编号 public: Vehicle(string no) { this->no = no; } virtual void display() = 0; //应收费用 }; class Car :public Vehicle { public: int num; float weight; Car(string no, int num, float weight) :Vehicle(no) { this->num = num; this->weight = weight; } void display() { cout << no << " " << num * 8 + weight + 2 << endl; } }; class Bus:public Vehicle { public: int num; Bus(string no, int num) :Vehicle(no) { this->num = num; } void display() { cout << no << " " << num*30 << endl; } }; class Truck :public Vehicle { public: float weight; Truck(string no, int weight) :Vehicle(no) { this->weight = weight; } void display() { cout << no << " " << weight* 5 << endl; } }; int main() { int t; cin >> t; int type; string no; int num; float weight; while (t--) { cin >> type >> no; if (type == 1){ cin >> num >> weight; Vehicle* pv = new Car(no,num,weight);; } else if (type == 2) { cin >> weight; Vehicle* pv = new Truck (no, weight);; } else if (type == 3) { cin >> num ; Vehicle* pv =new Bus(no,num);; } pv->display(); } return 0; }
时间: 2023-11-27 13:53:41 浏览: 65
在代码中,对象指针 `pv` 在条件语句中被定义并初始化,但是在条件语句块之外被使用。这会导致编译错误,因为在使用它时,它可能没有被初始化。要解决这个问题,可以将对象指针的定义移动到条件语句块之前。修改后的代码如下所示:
```
#include<iostream>
#include<string>
using namespace std;
class Vehicle {
protected:
string no; //编号
public:
Vehicle(string no)
{
this->no = no;
}
virtual void display() = 0; //应收费用
};
class Car :public Vehicle {
public:
int num;
float weight;
Car(string no, int num, float weight) :Vehicle(no)
{
this->num = num;
this->weight = weight;
}
void display()
{
cout << no << " " << num * 8 + weight + 2 << endl;
}
};
class Bus :public Vehicle {
public:
int num;
Bus(string no, int num) :Vehicle(no)
{
this->num = num;
}
void display()
{
cout << no << " " << num * 30 << endl;
}
};
class Truck :public Vehicle {
public:
float weight;
Truck(string no, float weight) :Vehicle(no)
{
this->weight = weight;
}
void display()
{
cout << no << " " << weight * 5 << endl;
}
};
int main() {
int t;
cin >> t;
int type;
string no;
int num;
float weight;
while (t--)
{
cin >> type >> no;
Vehicle* pv;
if (type == 1) {
cin >> num >> weight;
pv = new Car(no, num, weight);
}
else if (type == 2) {
cin >> weight;
pv = new Truck(no, weight);
}
else if (type == 3) {
cin >> num;
pv = new Bus(no, num);
}
pv->display();
}
return 0;
}
```
另外,还有一个小错误:在 `Truck` 类中的构造函数中,`weight` 参数应该为 `float` 类型而不是 `int` 类型。已经在上面的代码中进行了修正。
阅读全文
相关推荐
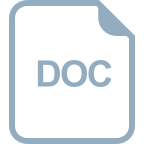
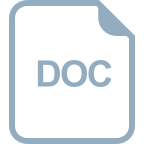
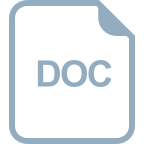


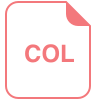












