python用get判断一个字典的键是否存在
时间: 2024-05-04 13:18:03 浏览: 16
可以使用 `get` 方法来判断一个字典的键是否存在,它的语法为 `字典.get(key, default=None)`,其中 `key` 为要查找的键,`default` 为键不存在时返回的默认值。如果字典中存在该键,则返回对应的值,否则返回默认值(如果指定了的话),或者返回 `None`。示例如下:
```python
my_dict = {"key1": "value1", "key2": "value2"}
# 判断键 key1 是否存在
if my_dict.get("key1") is not None:
print("key1 存在,对应的值为:", my_dict["key1"])
else:
print("key1 不存在")
# 判断键 key3 是否存在
if my_dict.get("key3") is not None:
print("key3 存在,对应的值为:", my_dict["key3"])
else:
print("key3 不存在")
```
输出结果为:
```
key1 存在,对应的值为: value1
key3 不存在
```
相关问题
python用if判断字典某个key值是否存在
可以使用 `in` 关键字来判断字典中是否存在某个 key 值,示例如下:
```python
my_dict = {'name': 'Alice', 'age': 18, 'gender': 'Female'}
if 'name' in my_dict:
print('name exists in the dictionary')
else:
print('name does not exist in the dictionary')
if 'height' in my_dict:
print('height exists in the dictionary')
else:
print('height does not exist in the dictionary')
```
输出结果为:
```
name exists in the dictionary
height does not exist in the dictionary
```
另外,也可以使用 `dict.get()` 方法来获取字典中指定 key 值的 value,如果该 key 值不存在,则返回 None。示例如下:
```python
my_dict = {'name': 'Alice', 'age': 18, 'gender': 'Female'}
name = my_dict.get('name')
print(name)
height = my_dict.get('height')
print(height)
```
输出结果为:
```
Alice
None
```
python返回字典某一个键用什么函数
在 Python 中,要返回字典中某一个键对应的值,可以使用 `dict[key]` 这样的方式。具体来说,你可以通过指定键名 `key` 来获取字典中对应的值。如果字典中不存在该键,则会抛出 `KeyError` 异常。
例如,假设有一个字典 `my_dict`,你想要获取键为 "name" 的值,可以使用以下方式:
```python
my_dict = {"name": "John", "age": 25, "city": "New York"}
value = my_dict["name"]
print(value) # 输出: John
```
请注意,在使用该方式时,确保字典中存在该键,否则会引发异常。如果你不确定键是否存在,可以使用 `dict.get(key, default)` 函数来获取值,并提供一个默认值 `default`,以防止出现键不存在的情况。例如:
```python
my_dict = {"name": "John", "age": 25, "city": "New York"}
value = my_dict.get("address", "Unknown")
print(value) # 输出: Unknown
```
在上述示例中,由于字典中不存在键为 "address" 的项,因此返回了提供的默认值 "Unknown"。
相关推荐
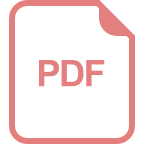
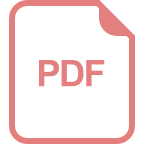












