java ftp上传大文件 并打印上传进度信息
时间: 2023-08-07 19:08:29 浏览: 208
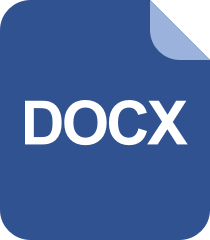
java ftp上传文件
好的,针对您的问题,我可以给你提供以下Java代码示例,用于FTP上传大文件并打印上传进度信息:
```
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
public class FTPUploader {
private FTPClient ftpClient;
public FTPUploader(String host, String user, String password) throws IOException {
ftpClient = new FTPClient();
ftpClient.connect(host);
ftpClient.enterLocalPassiveMode();
ftpClient.login(user, password);
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
}
public void uploadFile(String localFilePath, String remoteFilePath) throws IOException {
File localFile = new File(localFilePath);
BufferedInputStream inputStream = new BufferedInputStream(new FileInputStream(localFile));
ftpClient.storeFile(remoteFilePath, inputStream);
inputStream.close();
}
public void uploadFileWithProgress(String localFilePath, String remoteFilePath) throws IOException {
File localFile = new File(localFilePath);
BufferedInputStream inputStream = new BufferedInputStream(new FileInputStream(localFile));
long fileSize = localFile.length();
long uploadedSize = 0;
byte[] buffer = new byte[4096];
int bytesRead = -1;
while ((bytesRead = inputStream.read(buffer)) != -1) {
ftpClient.appendFile(remoteFilePath, new BufferedInputStream(new ByteArrayInputStream(buffer, 0, bytesRead)));
uploadedSize += bytesRead;
System.out.println("Uploaded " + uploadedSize + " bytes out of " + fileSize);
}
inputStream.close();
}
public void disconnect() throws IOException {
if (ftpClient.isConnected()) {
ftpClient.logout();
ftpClient.disconnect();
}
}
}
```
以上代码使用了 Apache Commons Net 库提供的 FTPClient 类,实现了 FTP 上传文件的功能。其中,uploadFile 方法用于上传小文件,而 uploadFileWithProgress 方法用于上传大文件并打印上传进度信息。
注意:在使用该代码时,需要将以下代码中的 `host`、`user` 和 `password` 替换为您的 FTP 服务器相关的信息。
```
FTPUploader uploader = new FTPUploader("host", "user", "password");
uploader.uploadFileWithProgress("local_file_path", "remote_file_path");
uploader.disconnect();
```
希望这个代码示例可以帮助到您。
阅读全文
相关推荐
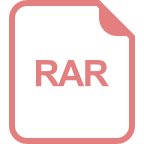

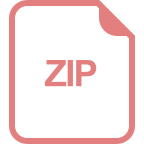
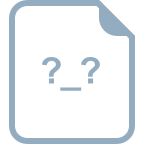
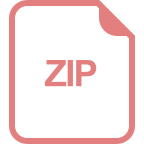
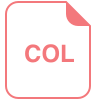
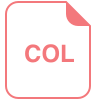
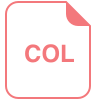
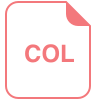
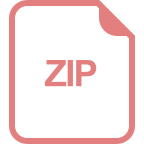
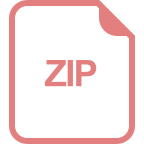