vue3怎么封装消息提醒组件,给出具体代码写法和用法
时间: 2024-05-15 13:12:59 浏览: 6
Vue3 中可以使用 Composition API 来封装消息提醒组件。下面是一个示例:
```vue
<template>
<div class="notification-container">
<div v-for="(notification, index) in notifications" :key="index" class="notification" :class="notification.type">
<div class="message">{{ notification.message }}</div>
<button @click="removeNotification(index)">x</button>
</div>
</div>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
const notifications = ref([]);
const addNotification = (message, type = 'info') => {
notifications.value.push({ message, type });
};
const removeNotification = (index) => {
notifications.value.splice(index, 1);
};
return {
notifications,
addNotification,
removeNotification,
};
},
};
</script>
<style>
.notification-container {
position: fixed;
bottom: 10px;
right: 10px;
z-index: 999;
}
.notification {
display: flex;
justify-content: space-between;
align-items: center;
padding: 10px;
margin-bottom: 10px;
border-radius: 5px;
color: #fff;
font-size: 14px;
}
.notification.info {
background-color: #2196f3;
}
.notification.success {
background-color: #4caf50;
}
.notification.warning {
background-color: #ffc107;
}
.notification.error {
background-color: #f44336;
}
.notification button {
background-color: transparent;
border: none;
color: inherit;
cursor: pointer;
font-size: 16px;
}
</style>
```
在上面的代码中,我们使用了 `ref` 创建了一个 `notifications` 变量,用于存储所有的消息提醒。我们还提供了两个方法 `addNotification` 和 `removeNotification`,用于添加和移除消息提醒。在模板中,我们使用 `v-for` 渲染出所有的消息提醒,并且使用 `:class` 来动态设置不同类型的样式。
使用该组件时,只需要在其他组件中导入该组件,并调用其 `addNotification` 方法即可:
```vue
<template>
<button @click="onClick">显示消息提醒</button>
</template>
<script>
import Notification from './Notification.vue';
export default {
components: {
Notification,
},
methods: {
onClick() {
this.$refs.notification.addNotification('这是一条普通的消息提醒');
this.$refs.notification.addNotification('这是一条成功的消息提醒', 'success');
this.$refs.notification.addNotification('这是一条警告的消息提醒', 'warning');
this.$refs.notification.addNotification('这是一条错误的消息提醒', 'error');
},
},
};
</script>
```
在上面的代码中,我们通过 `$refs` 获取了 `Notification` 组件的实例,并使用其 `addNotification` 方法添加了四条不同类型的消息提醒。
相关推荐
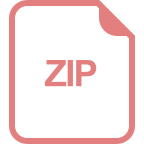
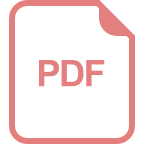
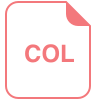
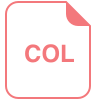












