c++输入一个文件名,程序查找是否存在此二进制数据文件,若不存在,则新建一个文件,若存在,则打开此文件并输出所有数据,提示用户输入若干个学生的学号和成绩,输入完成后将所有数据显示,并存入二进制数据文件
时间: 2023-12-05 10:03:26 浏览: 53
好的,你可以使用以下代码来实现这个功能:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
struct Student {
string id;
int score;
};
int main() {
string filename;
cout << "请输入文件名:";
cin >> filename;
ifstream fin(filename, ios::binary);
vector<Student> students;
if (fin) {
// 文件存在,读取数据
while (true) {
Student s;
fin.read(reinterpret_cast<char*>(&s), sizeof(Student));
if (fin.eof()) {
break;
}
students.push_back(s);
}
fin.close();
cout << "读取到的数据如下:" << endl;
for (const auto& s : students) {
cout << s.id << " " << s.score << endl;
}
} else {
// 文件不存在,创建文件
cout << "文件不存在,正在创建文件..." << endl;
}
// 输入学生信息
cout << "请输入若干个学生的学号和成绩(输入exit结束):" << endl;
while (true) {
string id;
int score;
cin >> id;
if (id == "exit") {
break;
}
cin >> score;
students.push_back({id, score});
}
// 输出学生信息并保存到文件
ofstream fout(filename, ios::binary);
for (const auto& s : students) {
fout.write(reinterpret_cast<const char*>(&s), sizeof(Student));
cout << s.id << " " << s.score << endl;
}
fout.close();
return 0;
}
```
该程序首先提示用户输入文件名,然后尝试打开该文件,如果文件存在,则读取其中的数据并输出;如果文件不存在,则创建一个新文件。然后程序提示用户输入若干个学生的学号和成绩,输入完成后将所有数据显示出来,并将数据保存到二进制数据文件中。注意,该程序使用了二进制文件读写方式,因此不能直接用文本编辑器打开和修改生成的数据文件。
相关推荐
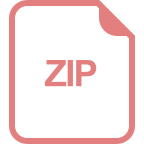
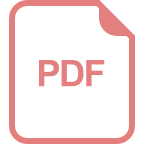
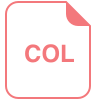
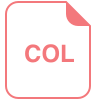

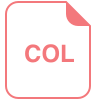
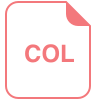









