检查图片是否损坏,修复后进行批量图片格式转换
时间: 2024-05-07 08:15:31 浏览: 190
以下是基于Python语言的代码实现:
```python
import os
from PIL import Image
# 检查图片是否损坏
def check_image(file_path):
try:
with Image.open(file_path) as img:
img.verify()
return True
except:
return False
# 修复图片
def fix_image(file_path, new_file_path):
with Image.open(file_path) as img:
img.load()
img.save(new_file_path)
# 批量图片格式转换
def convert_images(source_folder, target_folder, format):
if not os.path.exists(target_folder):
os.mkdir(target_folder)
for file_name in os.listdir(source_folder):
if file_name.endswith('.jpg') or file_name.endswith('.png'):
file_path = os.path.join(source_folder, file_name)
if check_image(file_path):
new_file_path = os.path.join(target_folder, file_name.split('.')[0] + '.' + format)
fix_image(file_path, new_file_path)
source_folder = 'path/to/source/folder'
target_folder = 'path/to/target/folder'
format = 'jpeg'
convert_images(source_folder, target_folder, format)
```
这段代码首先定义了三个函数,分别用于检查图片是否损坏、修复损坏的图片和进行批量图片格式转换。在批量图片格式转换函数中,我们首先检查目标文件夹是否存在,如果不存在则创建。然后遍历源文件夹中的所有图片文件,如果文件名以".jpg"或".png"结尾并且没有损坏,则进行格式转换。具体转换操作是,将原文件名的后缀改为目标格式的后缀,并将修复后的图片保存到目标文件夹中。
使用该代码时,只需将"path/to/source/folder"替换为实际的源文件夹路径,将"path/to/target/folder"替换为实际的目标文件夹路径,将"jpeg"替换为目标格式即可。
阅读全文
相关推荐
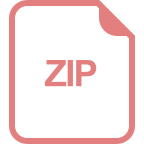
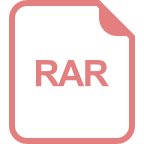
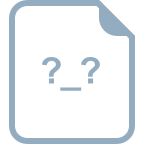
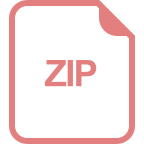
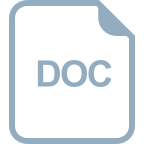
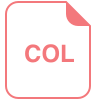
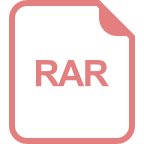
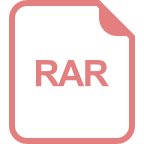
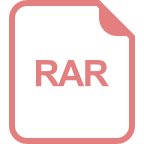
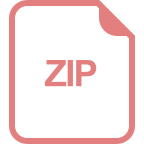
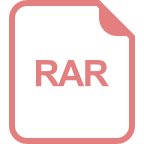
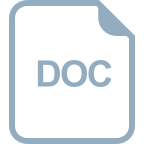
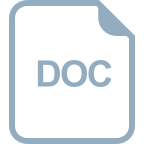
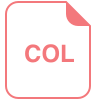
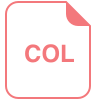
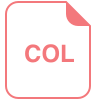
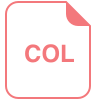