python可视化java ast
时间: 2024-06-08 07:07:34 浏览: 15
首先,AST(Abstract Syntax Tree)是一种数据结构,用于表示编程语言中的代码结构。在Python中,可以使用ast模块来生成Python代码的AST。而对于Java,也有相应的AST库可用,例如JavaParser和ANTLR等。
关于Python的AST可视化,可以使用Graphviz工具来将AST转换为图形化表示。具体实现可以参考以下代码:
```python
import ast
import graphviz
# 生成Python代码的AST
code = """
def foo(x, y):
z = x + y
return z
"""
tree = ast.parse(code)
# 定义节点颜色和形状
def get_node_attributes(node):
color = 'lightblue'
shape = 'box'
if isinstance(node, ast.Name):
color = 'lightpink'
elif isinstance(node, ast.Call):
color = 'lightgreen'
return {'color': color, 'shape': shape}
# 定义AST节点的标签
def label_node(node):
if isinstance(node, ast.AST):
fields = [(a, label_node(b)) for a, b in ast.iter_fields(node)]
return '%s\n%s' % (type(node).__name__, '\n'.join(('%s: %s' % field for field in fields)))
elif isinstance(node, list):
return '[' + ', '.join([label_node(x) for x in node]) + ']'
return str(node)
# 将AST转换为Graphviz图形
def ast2gv(node):
g = graphviz.Digraph()
def _ast2gv(node, parent):
attributes = get_node_attributes(node)
label = label_node(node)
g.node(str(id(node)), label=label, **attributes)
if parent is not None:
g.edge(str(id(parent)), str(id(node)))
for _, child in ast.iter_fields(node):
if isinstance(child, list):
for item in child:
if isinstance(item, ast.AST):
_ast2gv(item, node)
elif isinstance(child, ast.AST):
_ast2gv(child, node)
_ast2gv(node, None)
return g
# 显示图形
ast2gv(tree).view()
```
对于Java的AST可视化,可以使用ANTLR工具来获取Java代码的AST,并使用Graphviz工具进行图形化表示。具体实现可以参考以下步骤:
1. 安装ANTLR:将ANTLR下载到本地,并将antlr-x.x.jar文件添加到CLASSPATH中。
2. 定义Java语法规则:使用ANTLR语法规则定义Java代码的语法结构。
3. 生成Java代码的AST:使用ANTLR生成Java代码的AST,并将其保存到文件中。
4. 将AST转换为Graphviz图形:将AST文件读取到内存中,并使用Graphviz工具将其转换为图形化表示。
具体代码实现可以参考以下链接:https://github.com/antlr/grammars-v4/tree/master/java8/examples/Java2Dot。
总的来说,无论是Python还是Java,AST可视化都是一个非常有用的工具,可以帮助开发者更好地理解和分析代码结构。
相关推荐
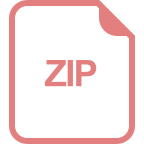
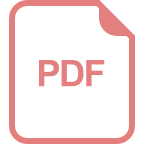














