vue生成一个抽奖转盘
时间: 2023-12-25 08:20:30 浏览: 41
生成抽奖转盘可以使用 Vue.js 和一些 JavaScript 库来实现。以下是一个简单的实现步骤:
1. 安装必要的库
使用 npm 安装以下库:
- vue:用于构建应用程序的主要框架。
- vue-router:用于创建 SPA 应用程序的路由库。
- axios:用于从 API 中获取数据的库。
- vue-axios:用于在 Vue 组件中使用 axios 的库。
- vue-luck-draw:用于创建抽奖转盘的库。
```
npm install vue vue-router axios vue-axios vue-luck-draw --save
```
2. 创建一个 Vue 组件
在 Vue 组件中,我们可以使用 vue-luck-draw 库创建一个抽奖转盘。以下是一个示例组件:
```
<template>
<div>
<vue-luck-draw :prizes="prizes" :buttons="buttons" :colors="colors" :bg-colors="bgColors" :is-start="isStart" :start-btn-text="startBtnText" :start-btn-color="startBtnColor" :start-btn-bg-color="startBtnBgColor" :start-btn-border-color="startBtnBorderColor" :speed="speed" :rotate-num="rotateNum" :rotate-end="rotateEnd" @start="onStart" @end="onEnd"></vue-luck-draw>
</div>
</template>
<script>
import VueLuckDraw from 'vue-luck-draw'
export default {
data() {
return {
prizes: [], // 奖品列表
buttons: [], // 按钮列表
colors: [], // 每个奖品扇形的颜色
bgColors: [], // 每个奖品扇形背景色
isStart: false, // 是否在进行抽奖
startBtnText: '开始', // 开始按钮文本
startBtnColor: '#ffffff', // 开始按钮文字颜色
startBtnBgColor: '#f00', // 开始按钮背景颜色
startBtnBorderColor: '#f00', // 开始按钮边框颜色
speed: 20, // 转盘速度
rotateNum: 8, // 转多少圈
rotateEnd: 0, // 转到哪个奖品位置
}
},
components: {
VueLuckDraw,
},
methods: {
onStart() {
// 抽奖开始
},
onEnd(prize) {
// 抽奖结束,prize 为中奖奖品
},
},
}
</script>
```
3. 设置奖品、按钮、颜色等参数
在组件中设置奖品、按钮、颜色等参数。以下是一个示例:
```
data() {
return {
prizes: [
{
id: 1,
name: '一等奖',
},
{
id: 2,
name: '二等奖',
},
{
id: 3,
name: '三等奖',
},
{
id: 4,
name: '谢谢参与',
},
{
id: 5,
name: '四等奖',
},
{
id: 6,
name: '五等奖',
},
],
buttons: [
{
id: 1,
name: '开始',
},
{
id: 2,
name: '重置',
},
],
colors: ['#ffff00', '#ff0000', '#ffff00', '#ff0000', '#ffff00', '#ff0000'],
bgColors: ['#ffffff', '#ffffff', '#ffffff', '#ffffff', '#ffffff', '#ffffff'],
}
},
```
4. 处理抽奖逻辑
在组件中处理抽奖逻辑。例如,在 onStart 方法中从后端 API 中获取中奖奖品,然后在 onEnd 方法中显示中奖信息。
```
methods: {
onStart() {
// 抽奖开始
this.isStart = true
// 从后端 API 中获取中奖奖品
axios.get('/api/prize')
.then(response => {
const prize = response.data.prize
// 计算中奖奖品位置
const index = this.prizes.findIndex(item => item.id === prize.id)
this.rotateEnd = (index + 1) * (360 / this.prizes.length) - 180 / this.prizes.length
// 停止转盘
setTimeout(() => {
this.isStart = false
}, 5000)
})
.catch(error => {
console.log(error)
})
},
onEnd(prize) {
// 抽奖结束,prize 为中奖奖品
alert(`恭喜您获得了${prize.name}`)
},
},
```
5. 添加样式
在组件中添加样式,例如:
```
<style scoped>
.vue-luck-draw {
width: 300px;
height: 300px;
margin: 0 auto;
}
</style>
```
6. 在路由中使用组件
在 Vue 路由中使用组件,例如:
```
import Vue from 'vue'
import Router from 'vue-router'
import Lottery from '@/components/Lottery'
Vue.use(Router)
export default new Router({
routes: [
{
path: '/',
name: 'Lottery',
component: Lottery,
},
],
})
```
7. 运行应用程序
运行应用程序,例如:
```
npm run serve
```
然后在浏览器中打开 http://localhost:8080。现在您应该可以看到一个抽奖转盘。
相关推荐
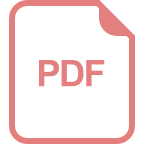














