give me an Python example using natural cubic spline
时间: 2024-05-09 11:21:31 浏览: 99
Sure, here's an example Python code for natural cubic spline:
```
import numpy as np
from scipy.interpolate import CubicSpline
# Define x and y values for data points
x = np.array([1, 2, 3, 4, 5])
y = np.array([0, 2, 1, 3, 2])
# Create natural cubic spline interpolator
cs = CubicSpline(x, y, bc_type='natural')
# Evaluate spline at a new point
new_x = 2.5
new_y = cs(new_x)
print('The value of the natural cubic spline at x = {} is y = {}'.format(new_x, new_y))
```
This code will create a natural cubic spline interpolator for the given x and y values, and then evaluate the spline at a new point (2.5 in this example). The output will be the value of the spline at that point.
相关问题
give me an Python example using natural cubic spline,do not use Scipy
Sure, here's an example of implementing natural cubic spline interpolation in Python without using Scipy:
```
import numpy as np
def natural_cubic_spline(x, y):
n = len(x)
a = y.copy()
b = np.zeros(n)
d = np.zeros(n)
h = np.diff(x)
alpha = np.zeros(n-1)
for i in range(1, n-1):
alpha[i] = 3*(a[i+1]-a[i])/h[i] - 3*(a[i]-a[i-1])/h[i-1]
l = np.zeros(n)
mu = np.zeros(n)
z = np.zeros(n)
l[0] = 1
mu[0] = z[0] = 0
for i in range(1, n-1):
l[i] = 2*(x[i+1]-x[i-1]) - h[i-1]*mu[i-1]
mu[i] = h[i]/l[i]
z[i] = (alpha[i]-h[i-1]*z[i-1])/l[i]
l[n-1] = 1
z[n-1] = c[n-1] = 0
for j in range(n-2, -1, -1):
c[j] = z[j] - mu[j]*c[j+1]
b[j] = (a[j+1]-a[j])/h[j] - h[j]*(c[j+1]+2*c[j])/3
d[j] = (c[j+1]-c[j])/(3*h[j])
return a, b, c, d
# Example usage
x = np.array([0, 1, 2, 3, 4])
y = np.array([0, 1, 2, 1, 0])
a, b, c, d = natural_cubic_spline(x, y)
print("Coefficients:")
print("a:", a)
print("b:", b)
print("c:", c)
print("d:", d)
```
give me an Python example using clamped cubic spline,do not use Scipy
Sure, here's an example Python code for clamped cubic spline without using Scipy:
```
import numpy as np
def clamped_cubic_spline(x, y, dydx0, dydxn):
n = len(x)
h = np.diff(x)
alpha = np.zeros(n)
l = np.ones(n)
u = np.zeros(n)
z = np.zeros(n)
for i in range(1, n - 1):
alpha[i] = (3 / h[i]) * (y[i+1] - y[i]) - (3 / h[i-1]) * (y[i] - y[i-1])
l[i] = 2 * (x[i+1] - x[i-1]) - h[i-1] * u[i-1]
u[i] = h[i] / l[i]
z[i] = (alpha[i] - h[i-1] * z[i-1]) / l[i]
b = np.zeros(n)
c = np.zeros(n)
d = np.zeros(n)
l[n-1] = 1
z[n-1] = 0
c[n-1] = 0
for j in range(n-2, -1, -1):
c[j] = z[j] - u[j] * c[j+1]
b[j] = (y[j+1] - y[j]) / h[j] - h[j] * (c[j+1] + 2 * c[j]) / 3
d[j] = (c[j+1] - c[j]) / (3 * h[j])
a = y[:-1]
b = b[:-1]
c = c[:-1]
d = d[:-1]
return a, b, c, d
x = np.array([0, 1, 2, 3, 4])
y = np.array([1, 2, 3, 2, 1])
dydx0 = 0
dydxn = 0
a, b, c, d = clamped_cubic_spline(x, y, dydx0, dydxn)
print("a =", a)
print("b =", b)
print("c =", c)
print("d =", d)
```
This code takes in arrays of `x` and `y` values, as well as the derivative values `dydx0` and `dydxn` at the endpoints. It outputs the coefficients `a`, `b`, `c`, and `d` of the clamped cubic spline. Note that this implementation assumes that the `x` values are evenly spaced.
阅读全文
相关推荐
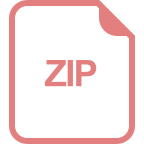
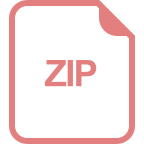
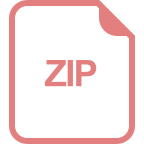


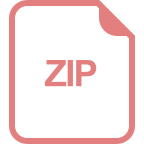
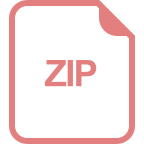
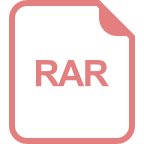
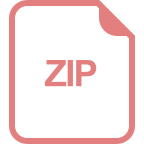
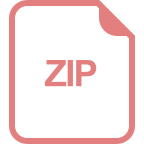
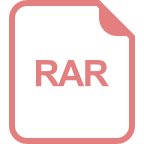
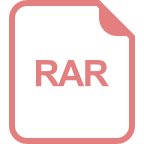
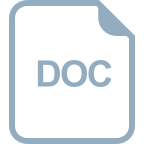


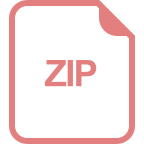