怎么只绘制上半图像的轮廓呢
时间: 2024-03-13 20:46:08 浏览: 7
可以使用 OpenCV 和 C++ 的语言特性,对轮廓点进行筛选,只保留 Y 坐标小于图像高度一半的轮廓点。下面是一个示例程序:
```cpp
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读取输入图像
Mat img = imread("input.png");
// 转换为灰度图像
Mat gray;
cvtColor(img, gray, COLOR_BGR2GRAY);
// 进行阈值化操作
Mat thresh;
threshold(gray, thresh, 127, 255, THRESH_BINARY);
// 查找轮廓
vector<vector<Point>> contours;
vector<Vec4i> hierarchy;
findContours(thresh, contours, hierarchy, RET_TREE, CHAIN_APPROX_SIMPLE);
// 筛选上半部分的轮廓点
int height = img.rows;
int half_height = height / 2;
vector<vector<Point>> filtered_contours;
for (const auto& contour : contours) {
vector<Point> filtered_contour;
for (const auto& point : contour) {
if (point.y < half_height) {
filtered_contour.push_back(point);
}
}
if (!filtered_contour.empty()) {
filtered_contours.push_back(filtered_contour);
}
}
// 在原图像上绘制轮廓
drawContours(img, filtered_contours, -1, Scalar(0, 0, 255), 2);
// 显示结果
imshow("Contours", img);
waitKey(0);
destroyAllWindows();
return 0;
}
```
在上面的代码中,我们首先读取输入图像并将其转换为灰度图像。然后使用 `cv::threshold()` 函数进行阈值化操作,将图像转换为二值图像。接下来使用 `findContours()` 函数查找图像中的轮廓。然后对每个轮廓点进行筛选,只保留 Y 坐标小于图像高度一半的点。最后将筛选后的轮廓绘制在原图像上,显示结果图像。
这个示例程序中,使用 `const auto&` 语法是为了避免不必要的拷贝,提高程序效率。
相关推荐
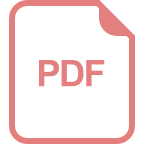
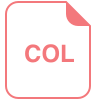
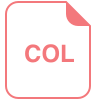











