用 Vue,手写一个轮播卡片叠加层叠滑动效果,卡牌动态滑动切换效果,不使用Swiper插件,移动端
时间: 2024-03-10 09:43:15 浏览: 141
好的,我会尽力回答你的问题。由于我是一个语言模型,无法展示效果图,但我可以提供代码实现思路和示例代码。
轮播卡片叠加层叠滑动效果:
1. 使用 Vue 组件实现每个卡片,并在样式中设置卡片的 z-index 和 transform 属性。
2. 给外层容器设置 overflow:hidden 属性,限制容器显示范围。
3. 使用 CSS3 动画实现卡片滑动效果。
4. 使用 Vue 监听 touch 事件,计算手指移动距离和速度,根据手指移动距离和速度计算卡片需要移动的距离和时间,然后使用 CSS3 动画实现卡片滑动效果。
示例代码:
```
<template>
<div class="card-container" @touchstart="onTouchStart" @touchmove="onTouchMove" @touchend="onTouchEnd">
<div class="card" v-for="(card, index) in cards" :key="index" :style="{ zIndex: (cards.length - index), transform: `translateX(${(index - currentCardIndex) * 100}%)` }"></div>
</div>
</template>
<style>
.card-container {
position: relative;
overflow: hidden;
height: 200px;
}
.card {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.2);
transition: all 0.3s ease;
}
.card:first-child {
transform: translateX(0);
}
.card:last-child {
transform: translateX(50%);
}
</style>
<script>
export default {
data() {
return {
cards: [1, 2, 3, 4, 5],
currentCardIndex: 0
}
},
methods: {
onTouchStart(e) {
// 记录手指起始位置
},
onTouchMove(e) {
// 计算手指移动距离和速度
// 根据手指移动距离和速度计算卡片需要移动的距离和时间
// 设置卡片 transform 属性实现滑动效果
},
onTouchEnd(e) {
// 根据手指移动距离和速度计算卡片需要移动的距离和时间
// 设置卡片 transform 属性实现滑动效果
}
}
}
</script>
```
卡牌动态滑动切换效果:
1. 使用 Vue 组件实现每个卡片,并在样式中设置卡片的宽度和 margin-right 属性。
2. 给外层容器设置 overflow:hidden 和 white-space: nowrap 属性,限制容器显示范围和让子元素在一行内显示。
3. 使用 CSS3 动画实现卡片滑动效果。
4. 使用 Vue 监听 touch 事件,计算手指移动距离和速度,根据手指移动距离和速度计算卡片需要移动的距离和时间,然后使用 CSS3 动画实现卡片滑动效果。
示例代码:
```
<template>
<div class="card-container" @touchstart="onTouchStart" @touchmove="onTouchMove" @touchend="onTouchEnd">
<div class="card" v-for="(card, index) in cards" :key="index"></div>
</div>
</template>
<style>
.card-container {
position: relative;
overflow: hidden;
white-space: nowrap;
height: 200px;
}
.card {
display: inline-block;
width: 80%;
height: 100%;
margin-right: 10%;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.2);
transition: all 0.3s ease;
}
.card:first-child {
margin-right: 0;
}
.card:last-child {
margin-right: 0;
}
</style>
<script>
export default {
data() {
return {
cards: [1, 2, 3, 4, 5],
currentCardIndex: 0
}
},
methods: {
onTouchStart(e) {
// 记录手指起始位置
},
onTouchMove(e) {
// 计算手指移动距离和速度
// 根据手指移动距离和速度计算卡片需要移动的距离和时间
// 设置卡片 transform 属性实现滑动效果
},
onTouchEnd(e) {
// 根据手指移动距离和速度计算卡片需要移动的距离和时间
// 设置卡片 transform 属性实现滑动效果
}
}
}
</script>
```
以上是代码实现思路和示例代码,你可以根据实际需求进行修改和优化。
阅读全文
相关推荐
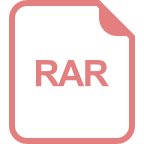
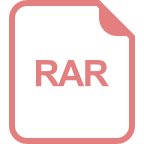

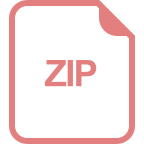
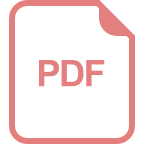
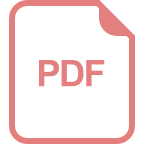
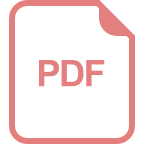
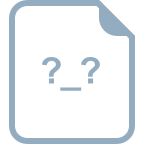
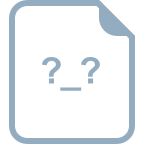
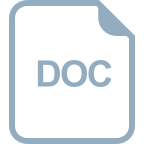
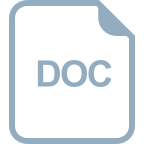






