用 Vue.js 写一个层叠卡片滑动切换、卡牌动态滑动切换效果,不适用插件
时间: 2024-03-09 12:51:03 浏览: 241
好的,下面是一个实现层叠卡片滑动切换、卡牌动态滑动切换效果的 Vue.js 实现示例:
HTML 代码:
```html
<div id="app">
<div class="swiper-container">
<div class="swiper-wrapper">
<div class="swiper-slide" v-for="(item, index) in cardList" :key="index">
<div class="card">
<div class="card-header">{{ item.title }}</div>
<div class="card-body">{{ item.content }}</div>
</div>
</div>
</div>
<div class="swiper-pagination"></div>
</div>
</div>
```
CSS 代码:
```css
.swiper-container {
width: 100%;
height: 300px;
position: relative;
}
.swiper-wrapper {
position: relative;
width: 100%;
height: 100%;
}
.swiper-slide {
position: absolute;
left: 0;
top: 0;
width: 100%;
height: 100%;
transition: transform 0.5s ease-in-out;
}
.swiper-pagination {
position: absolute;
bottom: 20px;
left: 50%;
transform: translateX(-50%);
}
.card {
background-color: #fff;
border: 1px solid #ccc;
border-radius: 5px;
box-shadow: 0 0 5px #ccc;
padding: 10px;
width: 80%;
margin: 0 auto;
}
.card-header {
font-weight: bold;
font-size: 18px;
}
.card-body {
margin-top: 10px;
}
```
JavaScript 代码:
```javascript
new Vue({
el: '#app',
data: {
currentIndex: 0,
cardList: [
{
title: 'Card Title 1',
content: 'Card Content 1'
},
{
title: 'Card Title 2',
content: 'Card Content 2'
},
{
title: 'Card Title 3',
content: 'Card Content 3'
},
{
title: 'Card Title 4',
content: 'Card Content 4'
},
{
title: 'Card Title 5',
content: 'Card Content 5'
}
]
},
mounted() {
this.initSwiper();
},
methods: {
initSwiper() {
const swiper = new Swiper('.swiper-container', {
loop: true,
centeredSlides: true,
slidesPerView: 'auto',
pagination: {
el: '.swiper-pagination',
clickable: true
},
on: {
slideChangeTransitionEnd: () => {
this.currentIndex = swiper.realIndex;
}
}
});
}
}
});
```
在这个示例中,我们使用了 Vue.js 和 Swiper 插件实现了层叠卡片滑动切换、卡牌动态滑动切换效果。具体来说,我们首先在 HTML 中创建了一个 `.swiper-container` 容器,然后在其中创建了若干个 `.swiper-slide` 卡片容器,每个卡片容器中包含了一个 `.card` 容器作为卡片的内容。我们使用了 Vue.js 的 `v-for` 指令动态渲染了卡片容器,并使用了 Swiper 的 `slidesPerView: 'auto'` 配置来实现卡片数量自适应。
在 JavaScript 中,我们使用了 Vue.js 的数据绑定机制来动态更新卡片容器的 `currentIndex` 属性,并在 Swiper 的 `slideChangeTransitionEnd` 事件中更新了 `currentIndex` 属性。最后,我们在 `mounted` 钩子函数中调用了 `initSwiper()` 方法来初始化 Swiper 插件。
阅读全文
相关推荐



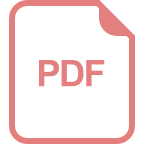
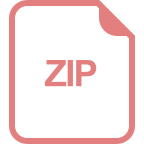
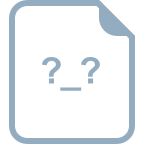
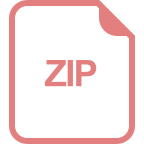
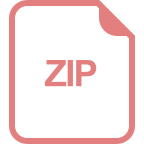
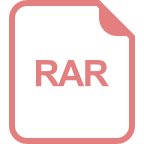
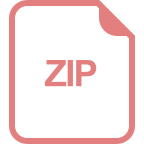
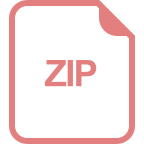
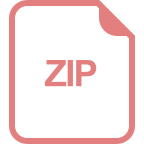
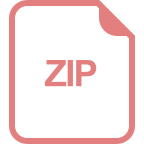
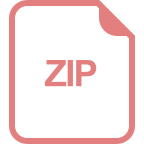
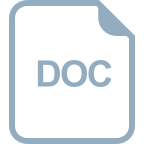
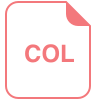