用 Vue,手写一个层叠卡片滑动切换、卡牌动态滑动切换效果,不使用Swiper插件
时间: 2024-03-09 12:51:11 浏览: 179
好的,下面是一个使用 Vue.js 手写实现层叠卡片滑动切换、卡牌动态滑动切换效果的示例:
HTML 代码:
```html
<div id="app">
<div class="card-container" ref="cardContainer">
<div class="card" v-for="(item, index) in cardList" :key="index" :style="{ zIndex: 100 - index, transform: 'translateX(' + (index - currentIndex) * 20 + 'px) scale(' + (10 - index) / 10 + ')' }">
<div class="card-header">{{ item.title }}</div>
<div class="card-body">{{ item.content }}</div>
</div>
</div>
<div class="pagination">
<span class="dot" v-for="(item, index) in cardList" :key="index" :class="{ active: index === currentIndex }" @click="handleDotClick(index)"></span>
</div>
</div>
```
CSS 代码:
```css
.card-container {
position: relative;
width: 100%;
height: 300px;
overflow: hidden;
}
.card {
position: absolute;
left: 50%;
transform-origin: center left;
width: 80%;
height: 80%;
background-color: #fff;
border: 1px solid #ccc;
border-radius: 5px;
box-shadow: 0 0 5px #ccc;
padding: 10px;
transition: transform 0.5s ease-in-out, z-index 0.5s;
}
.card-header {
font-weight: bold;
font-size: 18px;
}
.card-body {
margin-top: 10px;
}
.pagination {
margin-top: 20px;
text-align: center;
}
.dot {
display: inline-block;
width: 10px;
height: 10px;
margin: 0 5px;
border-radius: 50%;
background-color: #ccc;
cursor: pointer;
}
.dot.active {
background-color: #333;
}
```
JavaScript 代码:
```javascript
new Vue({
el: '#app',
data: {
currentIndex: 0,
cardList: [
{
title: 'Card Title 1',
content: 'Card Content 1'
},
{
title: 'Card Title 2',
content: 'Card Content 2'
},
{
title: 'Card Title 3',
content: 'Card Content 3'
},
{
title: 'Card Title 4',
content: 'Card Content 4'
},
{
title: 'Card Title 5',
content: 'Card Content 5'
}
]
},
mounted() {
this.$refs.cardContainer.addEventListener('mousedown', this.handleMouseDown);
this.$refs.cardContainer.addEventListener('mousemove', this.handleMouseMove);
this.$refs.cardContainer.addEventListener('mouseup', this.handleMouseUp);
this.$refs.cardContainer.addEventListener('mouseleave', this.handleMouseLeave);
},
destroyed() {
this.$refs.cardContainer.removeEventListener('mousedown', this.handleMouseDown);
this.$refs.cardContainer.removeEventListener('mousemove', this.handleMouseMove);
this.$refs.cardContainer.removeEventListener('mouseup', this.handleMouseUp);
this.$refs.cardContainer.removeEventListener('mouseleave', this.handleMouseLeave);
},
methods: {
handleDotClick(index) {
this.currentIndex = index;
},
handleMouseDown(event) {
this.mouseDown = true;
this.startX = event.clientX;
},
handleMouseMove(event) {
if (this.mouseDown) {
const offsetX = event.clientX - this.startX;
this.$refs.cardContainer.style.transform = 'translateX(' + (this.currentIndex * -20 + offsetX) + 'px)';
}
},
handleMouseUp(event) {
this.mouseDown = false;
const offsetX = event.clientX - this.startX;
const threshold = this.$refs.cardContainer.offsetWidth / 4;
if (offsetX > threshold && this.currentIndex > 0) {
this.currentIndex--;
} else if (offsetX < -threshold && this.currentIndex < this.cardList.length - 1) {
this.currentIndex++;
}
this.$refs.cardContainer.style.transform = 'translateX(' + (this.currentIndex * -20) + 'px)';
},
handleMouseLeave(event) {
this.mouseDown = false;
this.$refs.cardContainer.style.transform = 'translateX(' + (this.currentIndex * -20) + 'px)';
}
}
});
```
在这个示例中,我们手写实现了层叠卡片滑动切换、卡牌动态滑动切换效果,没有使用 Swiper 插件。具体来说,我们首先在 HTML 中创建了一个 `.card-container` 容器,然后在其中创建了若干个 `.card` 卡片容器,每个卡片容器中包含了一个 `.card-header` 和 `.card-body` 容器作为卡片的内容。我们使用了 Vue.js 的数据绑定机制动态渲染了卡片容器,并使用了 CSS3 的 `transform` 属性和 `z-index` 属性来实现层叠效果。
在 JavaScript 中,我们监听了鼠标的 `mousedown`、`mousemove`、`mouseup` 和 `mouseleave` 事件,并在 `handleMouseDown` 方法中记录了鼠标按下的起始位置,然后在 `handleMouseMove` 方法中根据鼠标移动的距离动态更新卡片容器的 `transform` 属性,实现卡片的动态滑动效果。在 `handleMouseUp` 方法中,我们根据鼠标移动的距离和速度判断当前应该显示哪一个卡片,并根据计算结果更新了 `currentIndex` 属性和卡片容器的 `transform` 属性。在 `handleMouseLeave` 方法中,我们重置了鼠标按下的状态和卡片容器的 `transform` 属性,保证了用户体验的连贯性。最后,我们在 `mounted` 钩子函数中添加了事件监听器,并在 `destroyed` 钩子函数中移除了事件监听器,以避免内存泄漏。
阅读全文
相关推荐
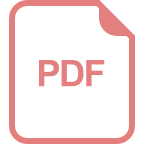



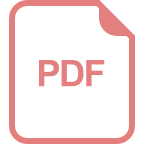
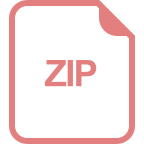
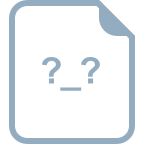
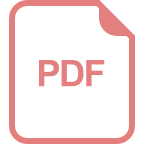
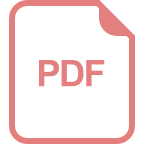
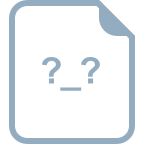
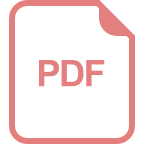
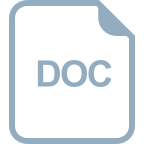
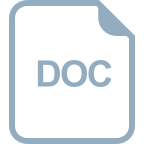



