写一个STM32f103c8t6的单通道、1kHz采样率的ADC程序,要求用定时器实现1khz采样率,并通过串口输出
时间: 2024-03-05 09:49:10 浏览: 129
好的,下面是一个基于STM32f103c8t6的单通道、1kHz采样率的ADC程序,通过定时器实现1kHz采样率,并通过串口输出ADC采样结果。
```c
#include "stm32f10x.h"
#include "stdio.h"
#define SAMPLE_RATE 1000 // 采样率为1kHz
#define BUFFER_SIZE 256 // 缓存区大小为256个采样点
uint16_t adc_buffer[BUFFER_SIZE]; // ADC采样结果缓存区
uint8_t buffer_index = 0; // 缓存区索引
USART_InitTypeDef USART_InitStructure;
void USART1_Init(void)
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void USART1_SendChar(uint8_t ch)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_TC) == RESET);
USART_SendData(USART1, ch);
}
void USART1_SendString(char* str)
{
while (*str) {
USART1_SendChar(*str++);
}
}
void TIM2_IRQHandler(void)
{
if (TIM_GetITStatus(TIM2, TIM_IT_Update) != RESET) {
ADC_SoftwareStartConvCmd(ADC1, ENABLE); // 触发ADC采样
while (ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC) == RESET); // 等待采样完成
adc_buffer[buffer_index++] = ADC_GetConversionValue(ADC1); // 存储采样结果
if (buffer_index >= BUFFER_SIZE) {
buffer_index = 0; // 缓存区溢出,重新开始
}
TIM_ClearITPendingBit(TIM2, TIM_IT_Update); // 清除定时器溢出中断标志
}
}
int main(void)
{
// 初始化ADC和定时器
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1 | RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AIN;
GPIO_Init(GPIOA, &GPIO_InitStructure);
ADC_InitTypeDef ADC_InitStructure;
ADC_InitStructure.ADC_Mode = ADC_Mode_Independent;
ADC_InitStructure.ADC_ScanConvMode = DISABLE;
ADC_InitStructure.ADC_ContinuousConvMode = DISABLE;
ADC_InitStructure.ADC_ExternalTrigConv = ADC_ExternalTrigConv_T2_CC2;
ADC_InitStructure.ADC_DataAlign = ADC_DataAlign_Right;
ADC_InitStructure.ADC_NbrOfChannel = 1;
ADC_Init(ADC1, &ADC_InitStructure);
ADC_RegularChannelConfig(ADC1, ADC_Channel_0, 1, ADC_SampleTime_239Cycles5);
ADC_Cmd(ADC1, ENABLE);
// 配置定时器的计数频率和自动重载值
uint16_t prescaler = SystemCoreClock / SAMPLE_RATE - 1;
uint16_t reload = 1000 - 1; // 定时器的计数频率为1kHz,自动重载值为1000-1
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
TIM_TimeBaseStructure.TIM_Period = reload;
TIM_TimeBaseStructure.TIM_Prescaler = prescaler;
TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure);
// 开启定时器中断
TIM_ITConfig(TIM2, TIM_IT_Update, ENABLE);
NVIC_EnableIRQ(TIM2_IRQn);
// 启动定时器
TIM_Cmd(TIM2, ENABLE);
// 初始化串口
USART1_Init();
while (1) {
// 定时读取缓存区中的ADC采样结果,并通过串口输出
for (int i = 0; i < BUFFER_SIZE; i++) {
char buffer[32];
sprintf(buffer, "%d\n", adc_buffer[i]);
USART1_SendString(buffer);
}
}
}
```
需要注意的是,这里只实现了通过串口输出ADC采样结果,如果需要进行其他处理和显示,可以在主程序中进行相应的修改和添加。另外,定时器的计数频率和自动重载值要根据系统时钟频率和采样率进行适当的计算和调整,以保证采样率的准确性和稳定性。
阅读全文
相关推荐

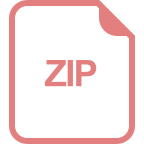
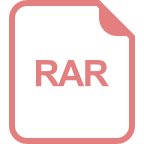
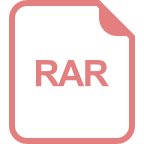
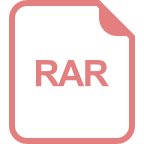
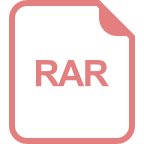
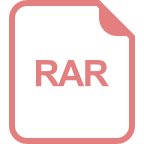
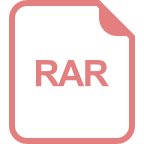
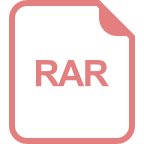
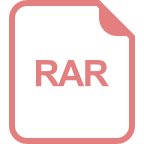
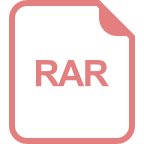