import itertools # 定义密码长度和字符集 length = 8 charset = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ' # 生成密码字典 passwords = [] for password in itertools.product(charset, repeat=length): password = ''.join(password) passwords.append(password) # 将密码字典保存到文件 with open('1.txt', 'w') as f: for password in passwords: f.write(1 + '\n')
时间: 2024-04-01 07:34:45 浏览: 32
这段代码看起来是一个密码字典生成器,使用了Python标准库itertools中的product函数来生成所有指定长度和字符集合的密码组合。然后将这些密码保存到文件中。不过,代码中最后一行有一个小错误,应该将数字1改为password。正确的代码如下:
```
import itertools # 定义密码长度和字符集 length = 8 charset = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ' # 生成密码字典 passwords = [] for password in itertools.product(charset, repeat=length): password = ''.join(password) passwords.append(password) # 将密码字典保存到文件 with open('1.txt', 'w') as f: for password in passwords: f.write(password + '\n')
```
相关问题
for i in itertools.product(data, repeat=password_length): guess = "".join(i)
### 回答1:
这段代码使用 Python 标准库中的 `itertools` 模块来生成指定长度 `password_length` 的所有可能组合,每个组合由 `data` 中的元素组成。然后通过 `join()` 方法将每个组合转换为字符串,赋值给变量 `guess`。这段代码通常被用于密码破解中的暴力破解攻击。但是需要注意,这种方法非常耗时,并且在实际应用中可能会受到各种限制,例如登录尝试次数限制等。因此,我们应该遵循安全最佳实践,使用强密码,并且定期更改密码。
### 回答2:
这段代码是使用itertools模块中的product函数来生成所有可能的组合。其中,data是一个给定的列表,password_length表示密码的长度。循环中,i代表product函数返回的一个个组合。通过"".join(i)将每个组合中的元素连接起来形成一个字符串,赋值给guess变量。
这段代码的作用是生成所有可能的密码猜测。假设data是一个包含数字和字母的列表,密码长度为4位,那么上述代码会生成包括数字和字母的所有4位组合的密码猜测。
举个例子,如果data是['1', '2', '3'],password_length为2,那么循环将生成6个密码猜测:'11'、'12'、'13'、'21'、'22'、'23'。
通过使用itertools.product函数生成所有可能的组合,并通过"".join函数将每个组合转换为字符串形式,可以方便地生成所有可能的密码猜测。但需要注意的是,随着data的大小和password_length的增加,生成的可能组合数量会呈指数级增长,可能需要很长的时间和资源来计算出所有的密码猜测。
import itertools# 定义多个数组array1 = [1, 2, 3, 4, 5]array2 = [2, 4, 6, 8, 10]array3 = [1, 3, 5, 7, 9]# 定义组合长度n = 3# 生成所有可能的组合combinations = itertools.combinations((array1, array2, array3), n)# 查找不重复的数字unique_numbers = set()for combination in combinations: flattened = list(itertools.chain.from_iterable(combination)) for number in flattened: if flattened.count(number) == 1: unique_numbers.add(number)# 输出结果print(unique_numbers)
这段代码的作用是从三个数组中生成所有长度为 3 的组合,然后找出这些组合中不重复的数字。具体实现如下:
1. 首先导入 itertools 模块,以便使用 combinations 函数来生成所有可能的组合。
2. 定义三个数组 array1、array2 和 array3,分别包含 5 个数字。
3. 定义变量 n,表示要生成的组合长度为 3。
4. 调用 itertools.combinations 函数来生成所有可能的组合,将其存储在变量 combinations 中。
5. 遍历所有组合,将其展开成一个一维列表 flattened,然后遍历该列表中的所有数字,如果该数字在列表中出现的次数为 1,则将其添加到集合 unique_numbers 中。
6. 最后输出集合 unique_numbers,即为不重复的数字。
需要注意的是,这段代码中的数组是硬编码在代码中的,如果需要处理其他的数组,需要手动修改代码中的数组。此外,由于该算法采用了嵌套循环,因此时间复杂度较高,在数组较大时可能会导致性能问题。
相关推荐
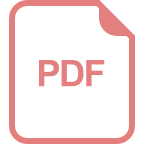
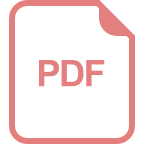
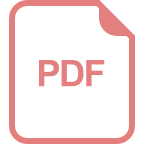












