learning_rate = list(itertools.product([0.001, 0.01, 0.1], repeat=2))
时间: 2024-05-23 22:13:42 浏览: 152
这行代码使用了 itertools 库中的 product() 函数,用于生成两个列表的笛卡尔积。在这里,第一个列表是 [0.001, 0.01, 0.1],第二个列表也是 [0.001, 0.01, 0.1]。repeat=2 意味着将这两个列表重复两次,因此生成的笛卡尔积将包含 9 个元素,即:
[(0.001, 0.001), (0.001, 0.01), (0.001, 0.1),
(0.01, 0.001), (0.01, 0.01), (0.01, 0.1),
(0.1, 0.001), (0.1, 0.01), (0.1, 0.1)]
这些元组可以用作学习率的组合,以便在机器学习模型中进行超参数调整。
相关问题
row_permutations = [itertools.permutations(row) for row in mp] matrix_permutations = itertools.product(*row_permutations)那这里的matrix_permutations呢,给我一些示例
`matrix_permutations = itertools.product(*row_permutations)` 会对 `row_permutations` 中的每个迭代器进行排列,然后将它们的笛卡尔积作为最终结果。因为 `row_permutations` 中的每个迭代器都是对应矩阵 `mp` 中的一行进行排列的,所以 `matrix_permutations` 中的每个元素都是一个排列好的矩阵。
以下是一个示例:
假设有一个矩阵 `mp`:
```
mp = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
```
使用 `row_permutations = [itertools.permutations(row) for row in mp]` 对每一行进行排列操作,并使用 `matrix_permutations = itertools.product(*row_permutations)` 对整个矩阵进行排列操作后,将得到以下 `matrix_permutations`:
```
[
((1, 2, 3), (4, 5, 6), (7, 8, 9)),
((1, 2, 3), (4, 5, 6), (7, 9, 8)),
((1, 2, 3), (4, 6, 5), (7, 8, 9)),
((1, 2, 3), (4, 6, 5), (7, 9, 8)),
((1, 3, 2), (4, 5, 6), (7, 8, 9)),
((1, 3, 2), (4, 5, 6), (7, 9, 8)),
((1, 3, 2), (4, 6, 5), (7, 8, 9)),
((1, 3, 2), (4, 6, 5), (7, 9, 8)),
((2, 1, 3), (4, 5, 6), (7, 8, 9)),
((2, 1, 3), (4, 5, 6), (7, 9, 8)),
((2, 1, 3), (4, 6, 5), (7, 8, 9)),
((2, 1, 3), (4, 6, 5), (7, 9, 8)),
((2, 3, 1), (4, 5, 6), (7, 8, 9)),
((2, 3, 1), (4, 5, 6), (7, 9, 8)),
((2, 3, 1), (4, 6, 5), (7, 8, 9)),
((2, 3, 1), (4, 6, 5), (7, 9, 8)),
((3, 1, 2), (4, 5, 6), (7, 8, 9)),
((3, 1, 2), (4, 5, 6), (7, 9, 8)),
((3, 1, 2), (4, 6, 5), (7, 8, 9)),
((3, 1, 2), (4, 6, 5), (7, 9, 8)),
((3, 2, 1), (4, 5, 6), (7, 8, 9)),
((3, 2, 1), (4, 5, 6), (7, 9, 8)),
((3, 2, 1), (4, 6, 5), (7, 8, 9)),
((3, 2, 1), (4, 6, 5), (7, 9, 8))
]
```
可以看到,这里的每个元素都是一个排列好的矩阵,其中第一个元素 `((1, 2, 3), (4, 5, 6), (7, 8, 9))` 就是原始矩阵 `mp`,而其他元素则是对 `mp` 进行不同排列得到的。
row_permutations = [itertools.permutations(row) for row in mp],row_permutations中排在前面的矩阵是哪些?给我一个实例
`row_permutations` 是一个列表,其中包含了每一行的排列结果。因为 `itertools.permutations(row)` 返回的是一个迭代器,所以 `row_permutations` 中每个元素也都是一个迭代器。
在 `row_permutations` 中,排在前面的矩阵是矩阵 `mp` 中的第一行对应的排列结果。以下是一个示例:
假设有一个矩阵 `mp`:
```
mp = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
```
使用 `row_permutations = [itertools.permutations(row) for row in mp]` 进行排列操作后,将得到以下 `row_permutations`:
```
[
<itertools.permutations object at 0x7fd1a3f6a7d0>,
<itertools.permutations object at 0x7fd1a3f6a810>,
<itertools.permutations object at 0x7fd1a3f6a850>
]
```
可以看到,`row_permutations` 中排在前面的迭代器是 `itertools.permutations(mp[0])`,也就是第一行 `[1, 2, 3]` 的排列结果。因为迭代器是惰性生成的,所以在这里并不会立即计算出所有的排列结果,而是在需要时才会生成。
如果想要查看第一行的排列结果,可以使用 `list` 函数将其转换为列表:
```
list(row_permutations[0])
```
输出结果为:
```
[(1, 2, 3), (1, 3, 2), (2, 1, 3), (2, 3, 1), (3, 1, 2), (3, 2, 1)]
```
可以看到,这里的第一个元素就是 `[1, 2, 3]` 的排列结果 `(1, 2, 3)`。
阅读全文
相关推荐
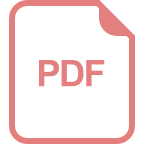
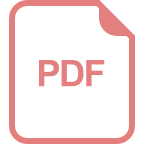
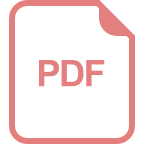













