python实现open3d绘制点和线组成的三维矩形框,并通过键盘控制矩形框移动
时间: 2023-03-31 08:02:53 浏览: 610
可以使用以下代码实现:
```python
import open3d as o3d
import numpy as np
# 创建点云
points = [[, , ], [1, , ], [1, 1, ], [, 1, ], [, , 1], [1, , 1], [1, 1, 1], [, 1, 1]]
lines = [[, 1], [1, 2], [2, 3], [3, ], [4, 5], [5, 6], [6, 7], [7, 4], [, 4], [1, 5], [2, 6], [3, 7]]
colors = [[1, , ] for i in range(len(lines))]
line_set = o3d.geometry.LineSet(points=o3d.utility.Vector3dVector(points), lines=o3d.utility.Vector2iVector(lines))
line_set.colors = o3d.utility.Vector3dVector(colors)
# 显示点云
o3d.visualization.draw_geometries([line_set])
# 通过键盘控制矩形框移动
def move_callback(vis):
# 获取当前矩形框的位置
current_pos = line_set.points
# 获取键盘事件
key = vis.get_key()
if key == ord('w'):
# 向前移动
current_pos[:, 2] += .1
elif key == ord('s'):
# 向后移动
current_pos[:, 2] -= .1
elif key == ord('a'):
# 向左移动
current_pos[:, ] -= .1
elif key == ord('d'):
# 向右移动
current_pos[:, ] += .1
# 更新矩形框的位置
line_set.points = o3d.utility.Vector3dVector(current_pos)
# 重新绘制点云
vis.update_geometry()
vis.poll_events()
vis.update_renderer()
# 注册键盘事件回调函数
o3d.visualization.draw_geometries_with_key_callbacks([line_set], {"w": move_callback, "s": move_callback, "a": move_callback, "d": move_callback})
```
阅读全文
相关推荐
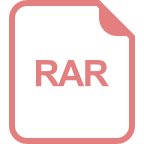
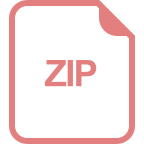
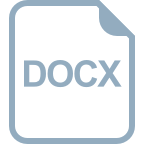
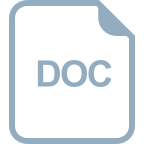
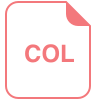
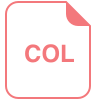
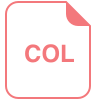
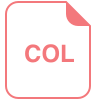
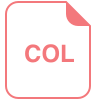
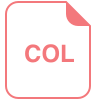
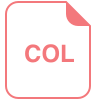
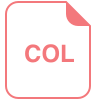
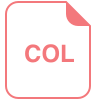
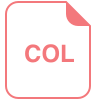
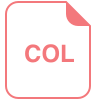
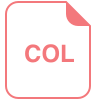
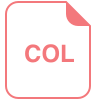