Python图形算法案例分析:解决实际问题的算法实现
发布时间: 2024-08-31 21:00:09 阅读量: 121 订阅数: 62 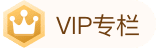
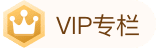
# 1. Python图形算法基础
在探索图形算法的世界时,Python作为一种强大的编程语言,提供了丰富的库和工具来处理和分析图形数据。本章旨在介绍Python中图形算法的基础知识,包括算法的定义、类型以及如何在Python环境下实现这些算法。我们将从最基础的图形理论开始,逐渐过渡到如何在Python中处理这些数据结构。通过本章的学习,读者将能够理解图形算法的基本概念,并具备初步的图形数据处理能力。接下来,我们将深入了解图形的基本理论,探索不同类型的图形算法,并讨论它们在现实世界应用中的重要性。
# 2. 图形算法的核心概念
图形算法是计算机科学和数学交叉领域的重要组成部分,它们广泛应用于数据结构、算法设计与分析、网络设计、资源分配等众多领域。理解图形算法的核心概念是构建图形应用和进行相关研究的前提。
### 图形的基本理论
图形(Graph)是由顶点(Vertex)集合和边(Edge)集合组成,用于表示对象之间关系的数据结构。在图形中,顶点可以类比为实体或对象,而边则表示实体间的某种关系或连接。图形分为无向图和有向图,其中无向图的边没有方向性,而有向图的边则有明确的方向。
- **无向图**:边不区分方向的图形。例如,社交网络中人与人之间的朋友关系可以表示为无向图。
- **有向图**:边有方向的图形。例如,网页之间的超链接关系可以构建为有向图,指向关系表示从一个网页跳转到另一个网页。
图形的表示方法主要有邻接矩阵和邻接表两种。邻接矩阵适用于边数较少的稠密图,而邻接表适合边数较多的稀疏图。
### 图形算法的分类
图形算法按照应用领域和处理功能可以分为多种类型:
- **路径算法**:寻找两个顶点之间的最短路径或最长路径。
- **网络流算法**:计算网络中最大流的大小,寻找最优的资源分配方案。
- **树算法**:包括生成树、最小生成树、最优二叉树等,用于优化网络结构。
- **匹配算法**:解决两组元素之间最优化的匹配问题。
## 图形算法在实际中的应用
图形算法在许多领域有广泛的应用,尤其在需要处理实体间关系和网络结构优化的场景中显得尤为重要。
### 设计领域的应用
在设计领域,图形算法可以用来分析和优化设计元素的布局和连接。例如,在电路板设计中,可以利用图形算法找到最小布线长度的解决方案,减少材料消耗并提高电路的可靠性。
### 游戏开发中的应用
在游戏开发中,图形算法用于构建复杂的游戏世界和角色互动。游戏内的地图通常可以表示为图形结构,使用图形算法可以有效地计算最短路径,实现AI角色的智能导航。此外,图形算法在游戏资源管理和优化中也起着关键作用。
### 机器视觉和模式识别
在机器视觉和模式识别中,图形算法用于表示和处理图像数据。通过将图像转换为图形结构,可以运用图形算法进行图像分割、边缘检测、特征提取等任务。图形神经网络(GNN)等现代技术更是直接利用图形算法处理复杂的数据结构。
## 算法效率分析
在设计和选择图形算法时,算法效率是一个关键考量因素。效率通常通过时间复杂度和空间复杂度来衡量。
### 时间复杂度和空间复杂度
时间复杂度表示算法执行时间随输入数据量的增加而变化的趋势。而空间复杂度则表示算法在运行过程中所占用的存储空间随输入数据量的增加而变化的趋势。
### 算法优化方法
为了提高图形算法的效率,可以采用多种优化方法,如剪枝技术、启发式搜索和并行处理等。剪枝技术通过剪除不可能产生最优解的分支来减少搜索空间。启发式搜索利用问题特定的知识来引导搜索过程,从而加快搜索速度。
### 示例代码分析
下面是一个简单的图遍历算法的示例代码,使用深度优先搜索(DFS)来遍历图中的所有顶点。我们将通过代码块逐行分析其逻辑。
```python
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start) # 可以执行其他操作,如输出顶点信息
for next in graph[start] - visited:
dfs(graph, next, visited)
return visited
# 示例图的邻接表表示
graph = {
'A': {'B', 'C'},
'B': {'A', 'D', 'E'},
'C': {'A', 'F'},
'D': {'B'},
'E': {'B', 'F'},
'F': {'C', 'E'}
}
# 执行深度优先搜索
dfs(graph, 'A')
```
- **第4行**:定义了一个函数`dfs`,它接受三个参数:图`graph`,开始顶点`start`,以及一个可选的`visited`集合来记录已访问顶点。
- **第6行**:如果`visited`没有提供,则初始化为一个空集合。
- **第7行**:将起始顶点添加到`visited`集合中,并输出顶点信息,可以根据实际需求修改此操作。
- **第9行**:遍历当前顶点`start`的所有邻接顶点。
- **第10行**:调用自身,递归地进行深度优先搜索。
此代码演示了深度优先搜索算法的基本实现和使用方法。通过执行这段代码,我们可以得到图的深度优先遍历顺序,并且能够直观地看到搜索过程中的顶点访问序列。
在实际应用中,深度优先搜索不仅可以用于遍历,还能用于其他很多方面,如检测环、拓扑排序等。理解其基本原理和使用方法是深入学习图形算法的基石。
# 3. Python中的图形绘制与处理
#### 3.1 Python图形库概览
Python拥有强大的图形库生态系统,为数据可视化和图像处理提供了丰富工具。在本节中,我们将探索两个最受欢迎的库:Matplotlib和Seaborn,它们分别提供基础和高级的数据可视化功能。
##### 3.1.1 Matplotlib的介绍与使用
Matplotlib是一个用于创建二维图表的库,包括线图、条形图、散点图、饼图等。它支持多种输出格式,如PDF、SVG、JPG、PNG等,并且可以嵌入到IPython记事本中,便于进行交互式数据可视化。
```python
import matplotlib.pyplot as plt
# 创建简单的线形图示例
plt.figure()
plt.plot([1, 2, 3, 4], [1, 4, 2, 3])
plt.title('Simple Plot')
plt.xlabel('X axis label')
plt.ylabel('Y axis label')
plt.show()
```
以上代码展示了一个基础的Matplotlib线形图绘制。首先导入`matplotlib.pyplot`模块,并调用`figure`函数创建图形窗口。通过`plot`函数绘制数据点,并使用`title`、`xlabel`、`ylabel`对图形进行标签设置。最后,`show`函数显示图形窗口。
##### 3.1.2 Seaborn的高级可视化功能
Seaborn是一个基于Matplotlib构建的高级可视化库,它提供了一系列简洁、美观的绘图选项,并且可以很方便地集成pandas数据结构
0
0
相关推荐
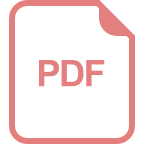
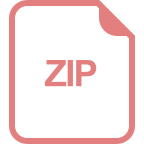
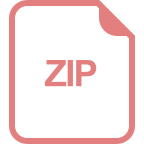





