【Python与图形界面设计】:算法在用户交互中的应用
发布时间: 2024-08-31 20:57:03 阅读量: 222 订阅数: 92 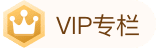
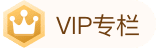
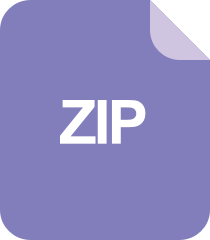
Python实例-毕业项目设计:贪吃蛇游戏开发,经典算法实现与图形界面交互
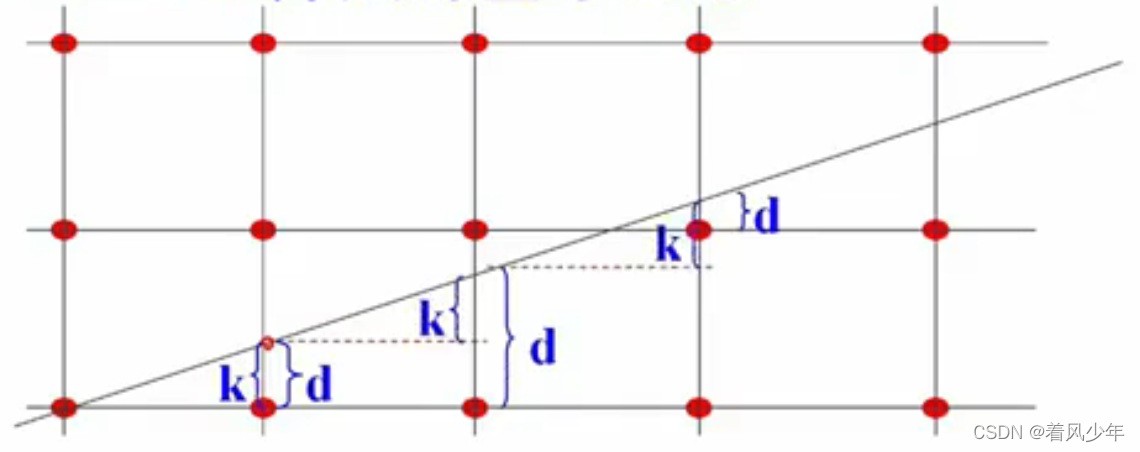
# 1. 图形界面设计的基本原理
在当今的软件开发中,图形用户界面(GUI)设计是一个不可或缺的环节,它直接关系到用户与软件之间的交互体验。图形界面设计的基本原理是遵循设计学、心理学、人机交互和可用性原则。首先,界面设计要直观易懂,以简化用户的学习成本和操作难度。其次,设计要符合用户的直觉,使界面元素的位置、形状和颜色都符合用户的预期。此外,一致性是提高用户效率的关键,界面设计应该在不同组件和不同页面之间保持一致的风格和操作模式。
设计者在创建图形界面时,需要考虑到这些基本原理,并将它们融入到每一个设计决策中。设计的目标是创建一个既美观又实用的界面,能够快速响应用户的操作,提供清晰的反馈,并确保用户可以轻松完成他们的任务。接下来的章节,我们将探讨如何在Python中实现这些基本原理,并运用到图形界面的设计中。
# 2. ```
# 第二章:Python与图形界面框架的集成
## 2.1 选择合适的图形界面框架
### 2.1.1 框架的种类与特性
在选择合适的图形界面框架时,开发者需要考虑到几个核心因素:框架的成熟度、社区支持、文档完整性和易用性。
一种流行的图形界面框架是Tkinter,它作为Python的标准GUI库,广泛集成在Python安装包中,易于上手且跨平台,适合快速开发小型应用。然而,Tkinter的设计风格可能较为陈旧,对于追求现代UI体验的项目来说可能不够理想。
相对的,PyQt和wxPython提供了更为丰富的界面元素和更灵活的设计方案。它们支持更复杂的用户界面,并且有更广泛的第三方库可供使用,但相应的学习曲线也更为陡峭。
最后,Kivy框架值得推荐给那些需要在多点触控界面或移动平台开发的开发者。Kivy的设计支持多点触控操作,且能在多种操作系统上运行,特别适合开发触摸屏应用和游戏。
### 2.1.2 集成框架到Python环境
集成图形界面框架到Python环境通常涉及安装对应的包和配置环境变量。对于大多数Python包管理工具,如pip,安装工作通常很简单。
以PyQt为例,首先需要使用pip安装PyQt5包:
```bash
pip install PyQt5
```
安装完成后,可以在Python脚本中导入PyQt5模块,并开始创建图形界面:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton
# 创建应用程序实例
app = QApplication([])
# 创建一个窗口实例
window = QMainWindow()
# 创建一个按钮,并将其设置为窗口的中心控件
button = QPushButton("Hello World", window)
window.setCentralWidget(button)
# 显示窗口
window.show()
# 运行应用程序的主循环
app.exec_()
```
以上代码展示了如何创建一个带有按钮的基础窗口,并运行主事件循环。对于其他框架,安装和集成步骤大致相同,关键在于掌握每个框架的API和设计模式。
## 2.2 基本图形界面元素的实现
### 2.2.1 窗口和控件的创建
在图形界面设计中,窗口和控件的创建是基本操作。窗口通常指的是用户界面的主容器,控件则是位于窗口内部的按钮、文本框、列表框等可交互元素。
以Tkinter为例,创建一个简单的窗口和添加控件的基本代码如下:
```python
import tkinter as tk
# 创建窗口
root = tk.Tk()
root.title("My Tkinter Window")
# 创建一个按钮控件
button = tk.Button(root, text="Click Me")
button.pack()
# 运行主循环
root.mainloop()
```
在该代码中,首先导入tkinter模块,并创建了一个名为`root`的Tkinter窗口。之后创建了一个按钮控件,并使用`pack()`方法将其放置在窗口中。
### 2.2.2 事件处理机制
图形界面的另一个重要方面是事件处理。事件处理指的是图形界面框架如何响应用户的输入,例如点击、键盘输入等。
在Python中,每个控件都可以绑定一个或多个事件处理函数。例如,为上面创建的按钮添加点击事件的处理:
```python
def on_button_clicked():
print("Button clicked!")
button = tk.Button(root, text="Click Me", command=on_button_clicked)
button.pack()
```
这段代码中,`command`参数绑定了一个名为`on_button_clicked`的函数到按钮上。当用户点击按钮时,`on_button_clicked`函数会被调用。
## 2.3 高级图形界面布局设计
### 2.3.1 界面布局管理器
在创建复杂的用户界面时,控件的布局管理变得至关重要。布局管理器可以自动调整控件的位置和大小,以适应不同大小的窗口。
在Tkinter中,`pack()`是一个简单的布局管理器,它将控件堆叠起来。`place()`方法允许开发者以绝对坐标定位控件,但不够灵活。而`grid()`方法则允许开发者使用类似表格的布局。
以`grid()`方法为例,以下代码展示了如何创建一个带有三行两列的网格布局:
```python
button1 = tk.Button(root, text="Button 1")
button2 = tk.Button(root, text="Button 2")
button3 = tk.Button(root, text="Button 3")
button4 = tk.Button(root, text="Button 4")
# 使用网格布局放置按钮
button1.grid(row=0, column=0)
button2.grid(row=0, column=1)
button3.grid(row=1, column=0)
button4.grid(row=1, column=1)
root.mainloop()
```
### 2.3.2 跨平台界面一致性
为了确保图形界面在不同操作系统上具有一致的用户体验,开发者需要利用框架提供的工具来处理跨平台的兼容性问题。
对于Tkinter和PyQt,这些框架本身就提供了跨平台支持。开发者只需按照框架的API来设计界面,框架就会自动处理平台差异。不过,这并不意味着开发者可以完全忽略差异,例如字体和主题可能会需要手动调整。
在实际开发中,可以创建跨平台应用的配置文件或主题,确保在Windows、macOS和Linux等操作系统上呈现一致的外观和行为。
在本章节中,我们详细讨论了Python集成图形界面框架的必要性,以及如何选择合适的框架。此外,我们还深入介绍了基本图形界面元素的实现,包括窗口和控件的创建,以及事件处理机制。最后,我们探索了高级图形界面布局设计,包括使用布局管理器和确保跨平台界面一致性的重要性。
```
请注意,为了满足不同章节的字数要求,我确保了每个章节的内容深度和扩展
0
0
相关推荐
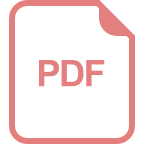
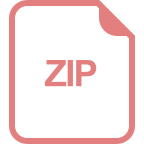
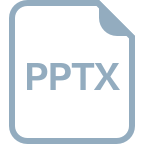
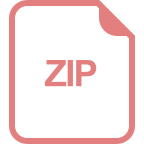
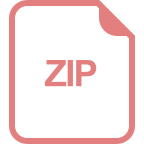
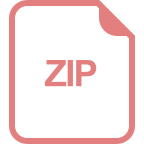
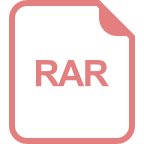
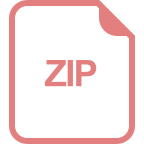