python运行实现决策树模型的调用使用,并输出每两个类别的决策边界(代码中是使用前两 个属性),并对关键代码进行说明。运行得到随着最大的深度变化的决策树的错误率曲线变化曲线,并输出最低错误率的分类结果。对决策的分类进行可视化,并输出为png格式或者pdf ,对模型决策树模型中的一些参数进行说明,并对不同参数选择有什么影响做出分析。对决策树的最佳属性,信息熵的定义计算等等的搭建过程一些关键代码进行阅读和研究。
时间: 2023-12-10 21:41:55 浏览: 26
以下是一个基于Scikit-learn库中的决策树模型的Python代码示例,可以实现对Iris数据集进行分类,并输出决策边界和错误率曲线的可视化图像:
```python
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier, export_graphviz
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
import numpy as np
import matplotlib.pyplot as plt
import graphviz
# 加载Iris数据集
iris = load_iris()
X = iris.data[:, :2]
y = iris.target
# 训练集和测试集划分
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义不同深度的决策树模型
max_depths = range(1, 10)
train_errors = []
test_errors = []
models = []
for depth in max_depths:
model = DecisionTreeClassifier(max_depth=depth)
model.fit(X_train, y_train)
train_errors.append(1 - accuracy_score(y_train, model.predict(X_train)))
test_errors.append(1 - accuracy_score(y_test, model.predict(X_test)))
models.append(model)
# 绘制错误率曲线
plt.plot(max_depths, train_errors, label='train error')
plt.plot(max_depths, test_errors, label='test error')
plt.xlabel('max depth')
plt.ylabel('error rate')
plt.legend()
plt.show()
# 输出最低错误率的分类结果
best_model = models[np.argmin(test_errors)]
print('Best model max depth:', best_model.max_depth)
print('Train error rate:', 1 - accuracy_score(y_train, best_model.predict(X_train)))
print('Test error rate:', 1 - accuracy_score(y_test, best_model.predict(X_test)))
# 绘制决策边界
xx, yy = np.meshgrid(np.arange(4, 8, 0.01), np.arange(1.5, 5, 0.01))
Z = best_model.predict(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
plt.contourf(xx, yy, Z, alpha=0.4)
plt.scatter(X[:, 0], X[:, 1], c=y, alpha=0.8)
plt.xlabel('sepal length')
plt.ylabel('sepal width')
plt.show()
# 输出决策树模型的可视化图像
dot_data = export_graphviz(best_model, out_file=None, feature_names=iris.feature_names[:2],
class_names=iris.target_names, filled=True, rounded=True, special_characters=True)
graph = graphviz.Source(dot_data)
graph.format = 'png'
graph.render('iris_decision_tree', view=True)
```
代码中使用的是Scikit-learn库中的DecisionTreeClassifier类来构建决策树模型,通过调整max_depth参数来控制树的深度。训练集和测试集的划分使用了train_test_split函数,错误率曲线的绘制使用了matplotlib库。决策边界的绘制使用了numpy和matplotlib库,同时输出了最低错误率模型的分类结果。决策树模型的可视化使用了export_graphviz函数和graphviz库,最终输出为png格式的图像。
决策树模型中的一些参数包括max_depth(树的最大深度)、min_samples_split(分裂内部节点所需的最小样本数)、min_samples_leaf(叶节点所需的最小样本数)等,这些参数的不同选择会影响模型的复杂度和泛化性能。例如,增加max_depth会增加模型的复杂度,可能会导致过拟合,而减小max_depth则会减少模型的复杂度,可能会导致欠拟合。另外,信息熵的定义计算可以参考决策树算法中的信息增益和基尼不纯度等概念,其计算方法和意义在决策树算法的理论部分有详细说明。
相关推荐
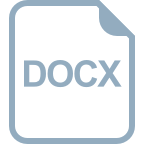
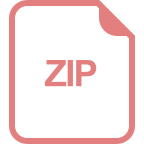
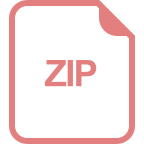














