叶片点云前后缘分割代码(c++)
时间: 2024-03-19 07:46:05 浏览: 19
以下是一个简单的基于欧几里得距离的叶片点云前后缘分割的 C++ 代码示例:
```
#include <iostream>
#include <vector>
#include <cmath>
using namespace std;
// 定义点云坐标结构体
struct Point {
float x, y, z;
};
// 计算欧几里得距离函数
float distance(Point p1, Point p2) {
return sqrt(pow(p1.x - p2.x, 2) + pow(p1.y - p2.y, 2) + pow(p1.z - p2.z, 2));
}
// 点云前后缘分割函数
void edgeDetection(vector<Point>& cloud, float threshold) {
for (int i = 0; i < cloud.size(); i++) {
float minDistance = threshold;
for (int j = 0; j < cloud.size(); j++) {
if (i != j) {
float dist = distance(cloud[i], cloud[j]);
if (dist < minDistance) {
minDistance = dist;
}
}
}
if (minDistance > threshold) {
cout << "Point (" << cloud[i].x << ", " << cloud[i].y << ", " << cloud[i].z << ") is an edge point." << endl;
}
}
}
// 主函数
int main() {
vector<Point> cloud = { {0, 0, 0}, {1, 0, 0}, {2, 0, 0}, {3, 0, 0}, {4, 0, 0}, {5, 0, 0}, {6, 0, 0}, {7, 0, 0},
{0, 1, 0}, {1, 1, 0}, {2, 1, 0}, {3, 1, 0}, {4, 1, 0}, {5, 1, 0}, {6, 1, 0}, {7, 1, 0},
{0, 2, 0}, {1, 2, 0}, {2, 2, 0}, {3, 2, 0}, {4, 2, 0}, {5, 2, 0}, {6, 2, 0}, {7, 2, 0},
{0, 3, 0}, {1, 3, 0}, {2, 3, 0}, {3, 3, 0}, {4, 3, 0}, {5, 3, 0}, {6, 3, 0}, {7, 3, 0},
{0, 4, 0}, {1, 4, 0}, {2, 4, 0}, {3, 4, 0}, {4, 4, 0}, {5, 4, 0}, {6, 4, 0}, {7, 4, 0},
{0, 5, 0}, {1, 5, 0}, {2, 5, 0}, {3, 5, 0}, {4, 5, 0}, {5, 5, 0}, {6, 5, 0}, {7, 5, 0},
{0, 6, 0}, {1, 6, 0}, {2, 6, 0}, {3, 6, 0}, {4, 6, 0}, {5, 6, 0}, {6, 6, 0}, {7, 6, 0},
{0, 7, 0}, {1, 7, 0}, {2, 7, 0}, {3, 7, 0}, {4, 7, 0}, {5, 7, 0}, {6, 7, 0}, {7, 7, 0} };
float threshold = 0.5;
edgeDetection(cloud, threshold);
return 0;
}
```
这个示例中,我们定义了一个 `Point` 结构体来存储点云中每个点的坐标,然后定义了一个 `distance` 函数来计算两个点之间的欧几里得距离。
接下来,我们实现了一个名为 `edgeDetection` 的函数,它接受一个点云向量和一个阈值作为参数。该函数通过计算每个点与其他点之间的距离来确定哪些点是边缘点,并将其打印到控制台上。
在主函数中,我们定义了一个简单的点云,并调用 `edgeDetection` 函数来查找前后缘点。
相关推荐
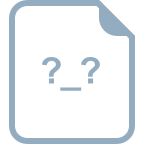
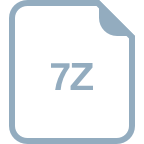














