taxi计费器c语言程序,基于单片机的出租车计价器的设计(附源程序)20130525.doc
时间: 2024-01-10 19:03:28 浏览: 100
很抱歉,我作为语言模型无法附上源程序,但我可以提供一些思路和思考方向。
要设计一个基于单片机的出租车计价器,我们需要考虑以下几个方面:
1. 计价规则
出租车计价规则一般是根据时间和里程计费,可以根据不同的时间段和里程数设置不同的费率。在程序中需要定义这些费率,并根据用户输入的里程数和时间计算出费用。
2. 输入和输出
计价器需要能够接收用户输入的里程数和时间,并输出计算结果。可以使用LCD屏幕显示计算结果,也可以使用蜂鸣器发出提示音。
3. 计算和存储
在程序中需要定义计算费用的算法,并将计算结果存储在单片机中。可以使用EEPROM或Flash存储。
4. 界面设计
出租车计价器的界面应该简洁明了,方便用户操作。可以使用按键输入里程数和时间,也可以使用旋转编码器调整数值。
以上是一些基本的思路和方向,具体的实现还需要结合硬件和具体的需求进行设计。
相关问题
vhdl出租车计价器设计
出租车计价器的设计可以使用 VHDL 语言来实现。以下是一种可能的设计方案:
1. 定义输入和输出端口:输入端口包括发车信号、上车信号、下车信号和计价器复位信号;输出端口包括计价器显示器的数码管和计价器的计费金额。
```
entity taxi_meter is
port (start: in std_logic;
get_on: in std_logic;
get_off: in std_logic;
reset: in std_logic;
fare: out std_logic_vector(15 downto 0);
displays: out std_logic_vector(6 downto 0));
end entity;
```
2. 定义内部信号:内部信号包括当前计费金额、计价器状态和数码管显示的值。
```
architecture rtl of taxi_meter is
signal current_fare: unsigned(15 downto 0) := (others => '0');
signal state: integer := 0;
signal display_value: std_logic_vector(6 downto 0) := "0000000";
begin
-- VHDL代码实现
end architecture;
```
3. 实现计价器状态机:根据输入信号和当前状态,计价器状态机可以转换到不同的状态,并且更新当前计费金额和数码管显示的值。
```
process(start, get_on, get_off, reset, current_fare, state)
begin
case state is
when 0 => -- 初始状态,等待发车信号
if start = '1' then
state <= 1;
end if;
when 1 => -- 发车状态,等待上车信号
if get_on = '1' then
state <= 2;
end if;
when 2 => -- 上车状态,等待下车信号或计价器复位信号
if get_off = '1' then
state <= 3;
elsif reset = '1' then
state <= 0;
current_fare <= (others => '0');
display_value <= "0000000";
end if;
when 3 => -- 下车状态,等待计价器复位信号
if reset = '1' then
state <= 0;
current_fare <= (others => '0');
display_value <= "0000000";
end if;
end case;
-- 更新计费金额和数码管显示的值
case state is
when 0 => -- 初始状态
display_value <= "0000000";
when 1 => -- 发车状态
current_fare <= (others => '0');
display_value <= "0000000";
when 2 => -- 上车状态
current_fare <= current_fare + 1;
display_value <= std_logic_vector(to_unsigned(current_fare, 7));
when 3 => -- 下车状态
current_fare <= current_fare + 2;
display_value <= std_logic_vector(to_unsigned(current_fare, 7));
end case;
end process;
```
4. 实现数码管显示模块:根据输入的数值,将其转换为数码管显示的格式。
```
process(display_value)
begin
case display_value is
when "0000000" => displays <= "1000000"; -- 显示0
when "0000001" => displays <= "1111001"; -- 显示1
when "0000010" => displays <= "0100100"; -- 显示2
when "0000011" => displays <= "0110000"; -- 显示3
when "0000100" => displays <= "0011001"; -- 显示4
when "0000101" => displays <= "0010010"; -- 显示5
when "0000110" => displays <= "0000010"; -- 显示6
when "0000111" => displays <= "1111000"; -- 显示7
when "0001000" => displays <= "0000000"; -- 显示8
when "0001001" => displays <= "0011000"; -- 显示9
when others => displays <= "1000000"; -- 显示0
end case;
end process;
```
5. 将计价器状态机和数码管显示模块组合在一起,完成整个出租车计价器的设计。
```
architecture rtl of taxi_meter is
signal current_fare: unsigned(15 downto 0) := (others => '0');
signal state: integer := 0;
signal display_value: std_logic_vector(6 downto 0) := "0000000";
begin
process(start, get_on, get_off, reset, current_fare, state)
begin
-- 计价器状态机的代码
end process;
process(display_value)
begin
-- 数码管显示模块的代码
end process;
-- 输出端口
fare <= std_logic_vector(current_fare);
displays <= display_value;
end architecture;
```
以上是一个简单的 VHDL 设计,仅供参考。实际的出租车计价器设计需要考虑更多的功能和细节,例如计价方式、计费规则、计价器显示格式等。
写一个基于VHDL的出租车计价器
以下是一个简单的基于VHDL的出租车计价器的代码实现:
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
entity taxi_meter is
Port ( clk : in STD_LOGIC;
reset : in STD_LOGIC;
start_trip : in STD_LOGIC;
stop_trip : in STD_LOGIC;
distance : in STD_LOGIC_VECTOR (7 downto 0);
fare : out STD_LOGIC_VECTOR (7 downto 0));
end taxi_meter;
architecture Behavioral of taxi_meter is
type state_type is (IDLE, TRIP_STARTED, TRIP_STOPPED);
signal state : state_type := IDLE;
signal total_distance : integer range 0 to 255 := 0;
signal total_fare : integer range 0 to 255 := 0;
constant fare_rate : integer := 10;
begin
process (clk, reset)
begin
if (reset = '1') then
state <= IDLE;
total_distance <= 0;
total_fare <= 0;
fare <= "00000000";
elsif (rising_edge(clk)) then
case state is
when IDLE =>
if (start_trip = '1') then
state <= TRIP_STARTED;
total_distance <= 0;
total_fare <= 0;
fare <= "00000000";
end if;
when TRIP_STARTED =>
if (stop_trip = '1') then
state <= TRIP_STOPPED;
total_fare <= total_distance * fare_rate;
fare <= std_logic_vector(to_unsigned(total_fare, 8));
else
total_distance <= total_distance + to_integer(unsigned(distance));
end if;
when TRIP_STOPPED =>
if (start_trip = '1') then
state <= TRIP_STARTED;
total_distance <= 0;
total_fare <= 0;
fare <= "00000000";
end if;
end case;
end if;
end process;
end Behavioral;
```
该计价器包含一个状态机,它可以在空闲、行程开始和行程结束之间转换。在行程开始状态下,计价器将累加距离并计算总费用。在行程结束状态下,计价器将输出最终费用并等待下一个行程的开始。
请注意,此代码仅用于演示目的,可能需要进行更多测试和验证才能用于实际应用。
阅读全文
相关推荐
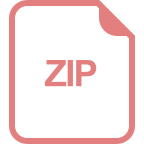
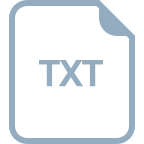
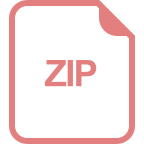













