c++友元运算符重载后为什么还是无法访问私有数据
时间: 2023-07-15 21:12:23 浏览: 130
重载运算符时,将其定义为友元函数可以让该运算符函数访问类的私有成员。但是,如果你在运算符函数中访问了类的私有数据,却仍然无法访问私有数据,可能是因为以下原因:
1. 友元函数没有正确声明到类中,如果友元函数没有正确声明到类中,那么它就无法访问类的私有成员。
2. 运算符函数参数类型不正确,运算符函数必须与类的参数匹配才能访问类的私有成员。
3. 运算符函数中使用了不同的类作为参数,如果你在运算符函数中使用了不同的类作为参数,那么它就无法访问当前类的私有成员。
请检查以上三个原因,如果都没有问题,那么你需要提供更多的细节信息,以便我们能更好地帮助你解决问题。
相关问题
c++,友元运算符重载例子
当然可以!C++是一种广泛使用的编程语言,它支持面向对象编程和运算符重载。友元(Friend)运算符重载是一种特殊的方法,允许友元函数或友元类访问类的私有和保护成员。下面是一个使用C++和友元运算符重载的简单例子:
```cpp
#include <iostream>
class MyClass {
private:
int x;
public:
// 构造函数
MyClass(int val) : x(val) {}
// 友元函数,用于输出成员变量x的值
friend void printValue(MyClass obj);
};
// 友元函数,访问类的私有成员x并输出其值
void printValue(MyClass obj) {
std::cout << "Value of x: " << obj.x << std::endl;
}
int main() {
MyClass obj(10); // 创建一个MyClass对象并初始化x为10
printValue(obj); // 调用友元函数,输出x的值
return 0;
}
```
在上面的例子中,我们定义了一个名为`MyClass`的类,它有一个私有成员变量`x`。我们使用友元函数`printValue`来访问和输出`x`的值。由于`printValue`是类的友元函数,它可以直接访问类的私有成员`x`。在主函数中,我们创建了一个`MyClass`对象并初始化`x`的值,然后调用友元函数`printValue`来输出`x`的值。
请注意,使用友元运算符重载需要谨慎,因为它可能会破坏封装性并导致代码难以维护。通常情况下,建议使用其他方法来实现运算符的重载,例如通过提供友元函数或非成员函数来实现相同的功能。
c++矩阵类及双目友元运算符重载
好的,针对您的问题,我可以给出以下代码示例:
```c++
#include <iostream>
#include <vector>
using namespace std;
class Matrix {
private:
int row, col;
vector<vector<int>> data;
public:
Matrix(int r, int c) : row(r), col(c), data(r, vector<int>(c)) {}
Matrix operator+(const Matrix& other) const {
if (row != other.row || col != other.col) {
throw runtime_error("Matrix dimensions do not match.");
}
Matrix res(row, col);
for (int i = 0; i < row; ++i) {
for (int j = 0; j < col; ++j) {
res.data[i][j] = data[i][j] + other.data[i][j];
}
}
return res;
}
Matrix operator*(const Matrix& other) const {
if (col != other.row) {
throw runtime_error("Matrix dimensions do not match.");
}
Matrix res(row, other.col);
for (int i = 0; i < row; ++i) {
for (int j = 0; j < other.col; ++j) {
for (int k = 0; k < col; ++k) {
res.data[i][j] += data[i][k] * other.data[k][j];
}
}
}
return res;
}
friend ostream& operator<<(ostream& os, const Matrix& mat) {
for (int i = 0; i < mat.row; ++i) {
for (int j = 0; j < mat.col; ++j) {
os << mat.data[i][j] << " ";
}
os << endl;
}
return os;
}
friend istream& operator>>(istream& is, Matrix& mat) {
for (int i = 0; i < mat.row; ++i) {
for (int j = 0; j < mat.col; ++j) {
is >> mat.data[i][j];
}
}
return is;
}
friend Matrix operator*(int scalar, const Matrix& mat) {
Matrix res(mat.row, mat.col);
for (int i = 0; i < mat.row; ++i) {
for (int j = 0; j < mat.col; ++j) {
res.data[i][j] = scalar * mat.data[i][j];
}
}
return res;
}
friend Matrix operator*(const Matrix& mat, int scalar) {
return scalar * mat;
}
friend Matrix operator-(const Matrix& mat) {
return -1 * mat;
}
friend Matrix operator-(const Matrix& lhs, const Matrix& rhs) {
return lhs + (-rhs);
}
friend bool operator==(const Matrix& lhs, const Matrix& rhs) {
if (lhs.row != rhs.row || lhs.col != rhs.col) {
return false;
}
for (int i = 0; i < lhs.row; ++i) {
for (int j = 0; j < lhs.col; ++j) {
if (lhs.data[i][j] != rhs.data[i][j]) {
return false;
}
}
}
return true;
}
friend bool operator!=(const Matrix& lhs, const Matrix& rhs) {
return !(lhs == rhs);
}
friend class BinoMatrix;
};
class BinoMatrix {
private:
Matrix data;
public:
BinoMatrix(int n) : data(n + 1, n + 1) {
for (int i = 0; i <= n; ++i) {
data.data[i][0] = 1;
for (int j = 1; j <= i; ++j) {
data.data[i][j] = data.data[i - 1][j - 1] + data.data[i - 1][j];
}
}
}
int operator()(int n, int k) const {
return data.data[n][k];
}
};
int main() {
Matrix mat1(2, 3), mat2(2, 3);
cin >> mat1 >> mat2;
cout << mat1 + mat2 << endl;
cout << mat1 - mat2 << endl;
cout << mat1 * mat2 << endl;
cout << 2 * mat1 << endl;
cout << mat1 * 2 << endl;
cout << -mat1 << endl;
cout << boolalpha << (mat1 == mat2) << endl;
cout << boolalpha << (mat1 != mat2) << endl;
BinoMatrix bino(10);
cout << bino(5, 2) << endl;
return 0;
}
```
这个示例代码实现了一个简单的矩阵类,支持加、减、乘、数乘、取负、相等性判断等运算,以及二项式系数的计算。其中,双目运算符重载使用了友元函数,以便访问私有成员变量。
阅读全文
相关推荐
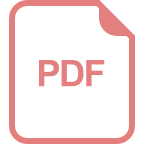
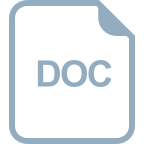





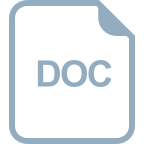
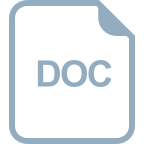
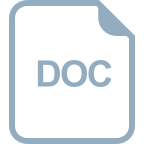
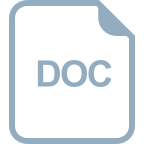
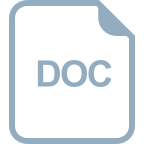
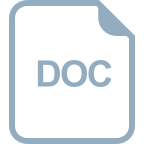
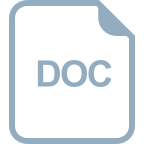
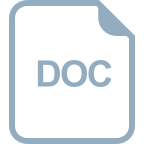