import numpy as np from sklearn.cluster import KMeans # 数据准备 data = np.array([ [100, 50, 20, 30, 80, 40, 10, 70], # 辽宁省的数据 [200, 150, 80, 60, 120, 100, 30, 90], # 浙江省的数据 [80, 60, 10, 20, 40, 30, 5, 50], # 河南省的数据 [50, 30, 5, 10, 25, 15, 3, 20], # 甘肃省的数据 [120, 80, 30, 40, 60, 50, 15, 40] # 青海省的数据 ]) # 数据标准化(可选,根据需要进行标准化处理) # data = (data - data.mean(axis=0)) / data.std(axis=0) # 使用K均值聚类算法进行分类 k = 5 # 分类的数量 kmeans = KMeans(n_clusters=k, random_state=0) labels = kmeans.fit_predict(data) # 打印分类结果 provinces = ["辽宁", "浙江", "河南", "甘肃", "青海"] for i, label in enumerate(labels): print(f"{provinces[i]}: 类别 {label}")生成相关图示
时间: 2023-07-02 11:08:33 浏览: 144
这段代码可以用来对省份数据进行 K 均值聚类,将省份划分为 k 个类别,并打印出每个省份所属的类别。
下面是一个简单的可视化示例,使用 matplotlib 库绘制一个散点图,不同颜色的点表示不同的类别,点的大小表示该省份的总数据量。代码如下:
```python
import matplotlib.pyplot as plt
# 绘制散点图
plt.scatter(data[:, 0], data[:, 1], s=data.sum(axis=1), c=labels)
plt.xlabel("数据1")
plt.ylabel("数据2")
plt.show()
```
其中,`data[:, 0]` 和 `data[:, 1]` 分别表示数据中的两个维度,`s=data.sum(axis=1)` 表示点的大小为该省份数据量的总和,`c=labels` 表示不同的类别用不同的颜色表示。
你可以根据需要修改代码,比如修改数据维度、调整点的大小和颜色等,以获得更好的可视化效果。
相关问题
import numpy as np from sklearn.cluster import KMeans def compress_image(image, n_clusters): # 转换为一维向量 data = image.reshape(-1, 3) # 用 KMeans 算法将像素点聚类到 n_clusters 个簇中 kmeans = KMeans(n_clusters=5, random_state=0).fit(data) # 将每个像素点替换为所属簇的中心像素值 compressed_data = np.array([kmeans.cluster_centers_[label] for label in kmeans.labels_]) # 将压缩后的一维向量转换回原图像的形状 compressed_image = compressed_data.reshape(image.shape) return compressed_imageimport matplotlib.pyplot as plt from PIL import Image # 读取图像 image = np.array(Image.open('0.jpg')) # 将图像数据归一化到 [0, 1] 范围内 image = image.astype('float32') / 255.0 # 压缩图像 compressed_image = compress_image(image_norm, n_clusters=16) # 显示压缩前后的图像 fig, ax = plt.subplots(1, 2, figsize=(10, 5)) ax[0].imshow(image) ax[0].set_title('Original Image') ax[1].imshow(compressed_image) ax[1].set_title('Compressed Image') plt.show()加上计算压缩率的功能
可以在 compress_image 函数中添加计算压缩率的功能,具体实现如下:
```
import numpy as np
from sklearn.cluster import KMeans
def compress_image(image, n_clusters):
# 转换为一维向量
data = image.reshape(-1, 3)
# 用 KMeans 算法将像素点聚类到 n_clusters 个簇中
kmeans = KMeans(n_clusters=n_clusters, random_state=0).fit(data)
# 将每个像素点替换为所属簇的中心像素值
compressed_data = np.array([kmeans.cluster_centers_[label] for label in kmeans.labels_])
# 将压缩后的一维向量转换回原图像的形状
compressed_image = compressed_data.reshape(image.shape)
# 计算压缩率
compressed_size = compressed_data.nbytes
original_size = image.nbytes
compression_ratio = compressed_size / original_size
return compressed_image, compression_ratio
import matplotlib.pyplot as plt
from PIL import Image
# 读取图像
image = np.array(Image.open('0.jpg'))
# 将图像数据归一化到 [0, 1] 范围内
image = image.astype('float32') / 255.0
# 压缩图像
n_clusters = 5
compressed_image, compression_ratio = compress_image(image, n_clusters)
# 显示压缩前后的图像
fig, ax = plt.subplots(1, 2, figsize=(10, 5))
ax[0].imshow(image)
ax[0].set_title('Original Image')
ax[1].imshow(compressed_image)
ax[1].set_title('Compressed Image')
# 显示压缩率
plt.figtext(0.5, 0.9, f'Compression Ratio: {compression_ratio:.2%}', ha='center', fontsize=12)
plt.show()
```
在上述代码中,计算压缩率的代码为:
```
compressed_size = compressed_data.nbytes
original_size = image.nbytes
compression_ratio = compressed_size / original_size
```
其中,`compressed_data.nbytes` 表示压缩后的图像数据的字节数,`image.nbytes` 表示原始图像数据的字节数,压缩率即为两者之比。
import pandas as pd import numpy as np from sklearn.cluster import KMeans import matplotlib.pyplot as plt def data_input(): data=pd.read_excel('22AI1.xlsx') data=data.dropna(axis=0) data = data.reset_index(drop=True) X=data.身高 Y=data.体重 X=np.array(X).reshape(-1,1) Y=np.array(Y).reshape(-1,1) return X,Y X,Y=data_input()[0],data_input()[1] X_trian=np.concatenate((X,Y),axis=1) clf=KMeans(n_clusters=3) clf.fit(X_trian) print(clf.labels_) plt.scatter(X,Y,c=clf.labels_) plt.show() ,报错ValueError: c of shape (35,) not acceptable as a color sequence for x with size 38, y with size 38
这个报错的原因是 `plt.scatter` 函数的参数 `c` 的形状与输入的 `X` 和 `Y` 的形状不相符。具体来说,`X` 和 `Y` 分别包含了 38 个身高和体重的数值,而 `clf.labels_` 包含了 35 个聚类标签。因此,你需要修改代码,使 `clf.labels_` 的形状与 `X` 和 `Y` 相同,可以通过将 `X` 和 `Y` 中的前 35 个元素与 `clf.labels_` 组合成一个新的数组来实现。修改后的代码如下所示:
```
import pandas as pd
import numpy as np
from sklearn.cluster import KMeans
import matplotlib.pyplot as plt
def data_input():
data=pd.read_excel('22AI1.xlsx')
data=data.dropna(axis=0)
data = data.reset_index(drop=True)
X=data.身高
Y=data.体重
X=np.array(X).reshape(-1,1)
Y=np.array(Y).reshape(-1,1)
return X,Y
X,Y=data_input()[0],data_input()[1]
X_train=np.concatenate((X,Y),axis=1)
clf=KMeans(n_clusters=3)
clf.fit(X_train)
labels = np.concatenate((clf.labels_, np.array([np.nan]*3)))
plt.scatter(X,Y,c=labels)
plt.show()
```
这样,就可以正确地显示聚类结果了。请注意,我在新的数组中添加了 3 个 `nan` 值,以便确保 `labels` 数组的长度与 `X` 和 `Y` 数组相同,并避免了 `plt.scatter` 函数的报错。
阅读全文
相关推荐
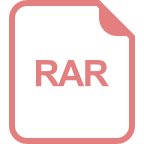
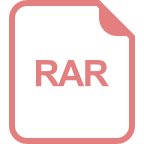
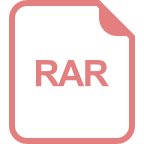












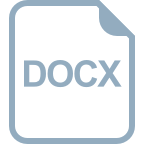