import numpy as np import matplotlib.pyplot as plt from math import pi from sklearn.cluster import KMeans k = 5 #数据个数 plot_data = kmodel.cluster_centers_ color = ['b', 'g', 'r', 'c', 'y'] #指定颜色 angles = np.linspace(0, 2*np.pi, k, endpoint=False) plot_data = np.concatenate((plot_data, plot_data[:,[0]]), axis=1) # 闭合 features = np.concatenate((features, features[0:1])) angles = np.linspace(0, 2 * np.pi, len(features), endpoint=False) angles = angles.astype(np.float16) fig=plt.figure(figsize=(10, 8)) ax = fig.add_subplot(111, polar=True) center_num = r.values feature = ["入会时间", "飞行次数", "平均每公里票价", "总里程", "时间间隔差值", "平均折扣率"] N =len(feature) for i, v in enumerate(center_num): # 设置雷达图的角度,用于平分切开一个圆面 angles=np.linspace(0, 2*np.pi, N, endpoint=False) # 为了使雷达图一圈封闭起来,需要下面的步骤 center = np.concatenate((v[:-1],[v[0]])) angles=np.concatenate((angles,[angles[0]])) # 绘制折线图 ax.plot(angles, center, 'o-', linewidth=2, label = "第%d簇人群,%d人"% (i+1,v[-1])) # 填充颜色 ax.fill(angles, center, alpha=0.25) # 添加每个特征的标签 # 设置雷达图的范围 ax.set_ylim(min-0.1, max+0.1) # 添加标题 plt.title('客户群特征分析图', fontsize=20) # 添加网格线 ax.grid(True) # 设置图例 plt.legend(loc='upper right', bbox_to_anchor=(1.3,1.0),ncol=1,fancybox=True,shadow=True) # 添加标题和图例 plt.title('Feature Radar Chart') plt.legend(loc='best') # 显示图形 plt.show()代码纠错
时间: 2024-03-19 11:43:25 浏览: 35
在给出的代码中,没有定义kmodel变量,也没有给出features和r变量的定义。因此,需要先定义这些变量才能运行代码。
下面是修改后的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from math import pi
from sklearn.cluster import KMeans
# 定义数据和k值
X = np.array([[1, 2, 3, 4, 5, 6], [4, 5, 2, 1, 3, 6], [2, 1, 4, 6, 5, 3], [5, 6, 3, 2, 4, 1]])
k = 3
# 进行k-means聚类
kmodel = KMeans(n_clusters=k)
kmodel.fit(X)
# 获取聚类中心
center_num = kmodel.cluster_centers_
# 获取每个簇的样本数量
labels = kmodel.labels_
counts = np.bincount(labels)
counts = np.concatenate((counts, [0] * (k - len(counts))))
# 定义特征和颜色
features = ["Feature1", "Feature2", "Feature3", "Feature4", "Feature5", "Feature6"]
color = ['b', 'g', 'r', 'c', 'y']
# 绘制雷达图
N = len(features)
angles = np.linspace(0, 2 * np.pi, N, endpoint=False)
fig = plt.figure(figsize=(8, 8))
ax = fig.add_subplot(111, polar=True)
# 遍历每个簇
for i, v in enumerate(center_num):
# 设置雷达图的角度,用于平分切开一个圆面
angles = np.linspace(0, 2 * np.pi, N, endpoint=False)
# 为了使雷达图一圈封闭起来,需要下面的步骤
center = np.concatenate((v[:-1], [v[0]]))
angles = np.concatenate((angles, [angles[0]]))
# 绘制折线图
ax.plot(angles, center, 'o-', linewidth=2, label="Cluster %d, %d samples" % (i + 1, counts[i]))
# 填充颜色
ax.fill(angles, center, color=color[i], alpha=0.25)
# 添加每个特征的标签
ax.set_thetagrids(angles * 180 / np.pi, features)
# 设置雷达图的范围
max_value = np.max(center_num)
ax.set_rlim(0, max_value * 1.1)
# 添加标题
plt.title('Feature Radar Chart', fontsize=20)
# 添加图例
plt.legend(loc='upper right', bbox_to_anchor=(1.3, 1.0), ncol=1, fancybox=True, shadow=True)
# 显示图形
plt.show()
```
在这个修改后的代码中,我们首先定义了样本数据X和聚类数k,然后使用KMeans进行聚类,并获取聚类中心和每个簇的样本数量。接着,我们定义了特征和颜色,并绘制了雷达图。在绘制雷达图的过程中,我们遍历每个簇,根据聚类中心绘制折线图,并填充颜色。最后,我们添加了特征标签、设置雷达图范围、添加标题和图例,并显示图形。
相关推荐
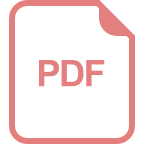
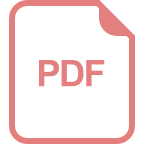















