string如何实现原子操作
时间: 2024-05-12 19:15:39 浏览: 4
在C++中,std::atomic<std::string>可以用来实现对std::string类型的原子操作。
使用std::atomic<std::string>时,需要注意以下几点:
1. 必须使用默认构造函数来初始化std::atomic<std::string>对象。
2. 对于std::string类型的原子操作,只能使用std::atomic<std::string>提供的一些特殊的操作函数,如load(), store(), exchange()等。
3. 由于std::string类型是动态分配的,所以需要使用std::shared_ptr<std::string>来进行管理。
下面是一个简单的示例代码,展示了如何使用std::atomic<std::string>实现对std::string类型的原子操作:
```c++
#include <iostream>
#include <thread>
#include <atomic>
#include <string>
#include <memory>
std::atomic<std::shared_ptr<std::string>> atomic_str;
void write_thread() {
std::shared_ptr<std::string> new_str(new std::string("Hello"));
atomic_str.store(new_str);
}
void read_thread() {
std::shared_ptr<std::string> str_ptr = atomic_str.load();
std::cout << *str_ptr << std::endl;
}
int main() {
std::thread t1(write_thread);
std::thread t2(read_thread);
t1.join();
t2.join();
return 0;
}
```
在上面的示例中,我们使用了std::atomic<std::shared_ptr<std::string>>来实现对std::string类型的原子操作。在write_thread()函数中,我们创建了一个新的std::string对象,并将其包装在std::shared_ptr中。然后,我们使用std::atomic<std::shared_ptr<std::string>>的store()函数将其存储到atomic_str对象中。
在read_thread()函数中,我们使用std::atomic<std::shared_ptr<std::string>>的load()函数获取atomic_str对象中存储的std::shared_ptr<std::string>指针,并通过该指针访问std::string对象。
值得注意的是,由于std::string类型是动态分配的,所以我们需要使用std::shared_ptr<std::string>来进行管理。这可以确保在多个线程之间安全地共享std::string对象。
相关推荐
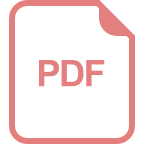
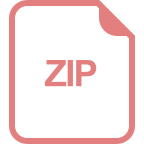
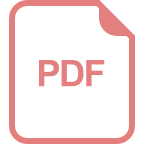
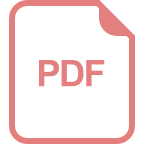
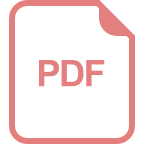
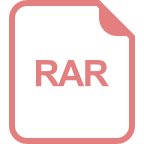
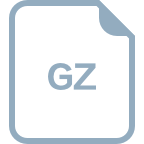
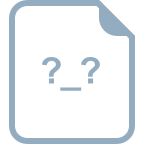
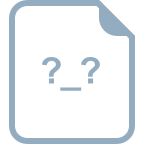
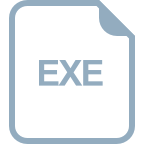
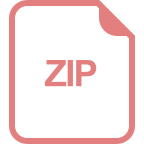
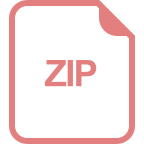
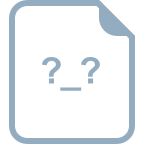
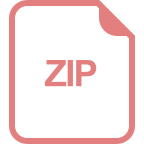