使用AES对压缩文件里的文件加密的python代码
时间: 2024-06-12 08:04:14 浏览: 4
以下是一个使用AES对压缩文件里的文件加密的Python代码示例:
```
import os
import zipfile
from Crypto.Cipher import AES
def encrypt_file(key, in_filename, out_filename=None, chunksize=64*1024):
if not out_filename:
out_filename = in_filename + '.enc'
iv = os.urandom(16)
encryptor = AES.new(key, AES.MODE_CBC, iv)
with open(in_filename, 'rb') as infile:
with open(out_filename, 'wb') as outfile:
outfile.write(iv)
while True:
chunk = infile.read(chunksize)
if len(chunk) == 0:
break
elif len(chunk) % 16 != 0:
chunk += b' ' * (16 - len(chunk) % 16)
outfile.write(encryptor.encrypt(chunk))
def encrypt_zipfile(key, in_filename, out_filename=None, chunksize=64*1024):
if not out_filename:
out_filename = in_filename + '.enc'
iv = os.urandom(16)
encryptor = AES.new(key, AES.MODE_CBC, iv)
with zipfile.ZipFile(in_filename, 'r') as inzip:
with zipfile.ZipFile(out_filename, 'w') as outzip:
for filename in inzip.namelist():
with inzip.open(filename, 'r') as infile:
with outzip.open(filename, 'w') as outfile:
outfile.write(iv)
while True:
chunk = infile.read(chunksize)
if len(chunk) == 0:
break
elif len(chunk) % 16 != 0:
chunk += b' ' * (16 - len(chunk) % 16)
outfile.write(encryptor.encrypt(chunk))
# Example usage
key = b'mysecretkey12345'
encrypt_file(key, 'mydata.txt', 'mydata.txt.enc')
encrypt_zipfile(key, 'mydata.zip', 'mydata.zip.enc')
```
该代码使用了PyCrypto库中的AES模块进行加密,同时使用了Python内置的zipfile模块来处理压缩文件。加密过程中,每个文件都会使用一个随机的初始化向量(IV)来增加安全性。加密后的文件会在原文件名后加上“.enc”后缀。注意,这里只是对文件进行了加密,如果需要对整个压缩文件进行加密,则需要先解压缩文件,然后对每个文件进行加密,最后再进行压缩。
相关推荐
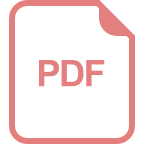
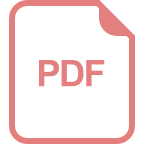
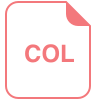
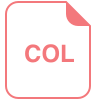
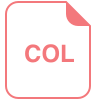
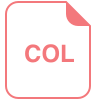
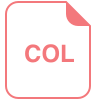









