分析此段代码功能: from sklearn.datasets import load_boston boston=load_boston() boston.data.shape
时间: 2023-12-26 15:05:08 浏览: 94
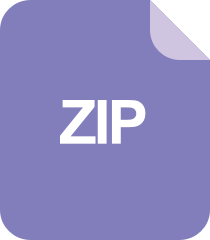
Boston-Model-Housing-prices-Multiple-Regression:使用多元回归模型从sklearn.datasets.load_boston预测房价
这段代码的功能是导入scikit-learn库中的load_boston方法,用于加载波士顿房价数据集,并将数据集存储在变量boston中。最后,该代码返回数据集的维度,即数据集包含的样本数和特征数。其中,boston.data.shape用于返回数据集的维度信息,也就是该数据集包含的样本数和特征数。
阅读全文
相关推荐
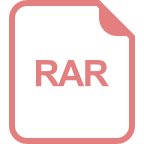
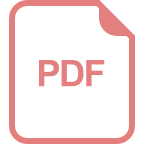


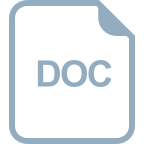
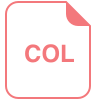
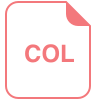










