调用函数fun,功能是用选择法对10个整数按降序排序
时间: 2023-11-21 18:59:32 浏览: 36
下面是一个用选择排序算法对一个长度为10的整数数组进行降序排序的示例代码:
```python
def fun(arr):
for i in range(len(arr)):
max_index = i
for j in range(i+1, len(arr)):
if arr[j] > arr[max_index]:
max_index = j
arr[i], arr[max_index] = arr[max_index], arr[i]
return arr
```
在函数中,我们首先使用一个外层循环来遍历整个数组,并使用一个变量`max_index`来记录当前未排序部分中最大元素的索引。然后,我们使用一个内层循环来查找未排序部分中的最大元素,并将其索引赋值给`max_index`。在内层循环结束后,我们将未排序部分中的最大元素与当前位置的元素交换。最终,我们将排序后的数组返回给调用者。
你可以将这个函数用于任何长度为10的整数数组。例如,如果你有一个名为`arr`的数组,你可以这样调用函数:
```python
arr = [3, 7, 1, 9, 4, 6, 2, 8, 5, 0]
sorted_arr = fun(arr)
print(sorted_arr)
```
输出将是`[9, 8, 7, 6, 5, 4, 3, 2, 1, 0]`,表示数组已经按降序进行了排序。
相关问题
c语言调用函数fun其功能是用选择法对10个整数按降序排序
以下是一个使用选择排序算法对10个整数按降序排序的C语言函数fun的实现:
```c
void fun(int arr[], int n) {
int i, j, temp, max_index;
for (i = 0; i < n - 1; i++) {
max_index = i;
for (j = i + 1; j < n; j++) {
if (arr[j] > arr[max_index]) {
max_index = j;
}
}
if (max_index != i) {
temp = arr[i];
arr[i] = arr[max_index];
arr[max_index] = temp;
}
}
}
```
该函数的参数包括一个包含10个整数的数组和数组的长度n。在函数内部,我们使用选择排序算法对数组进行降序排序。首先,我们从数组的第一个元素开始,找到数组中最大的元素,并将其与第一个元素交换。然后,我们从第二个元素开始,找到剩余元素中最大的元素,并将其与第二个元素交换。重复此过程,直到整个数组被排序。
使用c语言调用函数fun,功能是用选择法对10个整数按降序排序
以下是使用选择排序算法对10个整数进行降序排序的C语言代码:
```c
#include <stdio.h>
// 函数声明
void fun(int arr[], int n);
int main() {
int arr[10] = { 9, 6, 3, 7, 5, 2, 8, 1, 4, 0 };
int n = 10;
printf("排序前:");
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
fun(arr, n);
printf("\n排序后:");
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
return 0;
}
// 函数定义
void fun(int arr[], int n) {
int temp;
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (arr[i] < arr[j]) {
temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
}
}
```
输出结果:
```
排序前:9 6 3 7 5 2 8 1 4 0
排序后:9 8 7 6 5 4 3 2 1 0
```
相关推荐
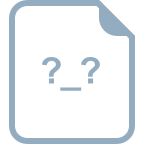
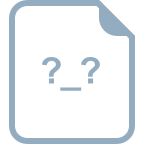
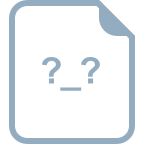












