ubuntu dlib c++
时间: 2023-05-04 16:06:47 浏览: 193
Ubuntu是一款基于Debian Linux的操作系统,它具有方便易用、安全、可靠和强大的特点。它可以在桌面和服务器上使用,并且它的软件库非常丰富。用户可以通过终端或GUI来控制Ubuntu系统。
dlib是一种面向机器学习的C++库,它包含许多用于分类、回归、聚类和图像处理的算法。该库的设计非常灵活,易于扩展,因此可以很容易地将其用于各种应用程序中。
C语言是一种通用、高级的编程语言,它被广泛应用于各种系统和应用程序中。它是一种结构化语言,因此程序员可以将代码划分为可重用的块,从而提高代码的可读性和可维护性。
将Ubuntu、dlib和C语言结合使用可以实现很多有用的应用程序。例如,可以使用dlib库训练和部署人脸识别系统,然后在Ubuntu操作系统上运行该系统。由于Ubuntu具有强大的系统管理和安全性,因此可以确保该系统的高效性和安全性。此外,由于C语言在速度和内存使用方面具有很高的效率,因此可以在Ubuntu上使用它来编写高性能的应用程序。
相关问题
C++如何使用opencv和dlib实现人脸识别
要实现人脸识别,需要使用OpenCV和Dlib这两个库。以下是使用C++实现人脸识别的基本步骤:
1. 安装OpenCV和Dlib库
首先需要安装OpenCV和Dlib库,并将其包含到C++项目中。可以使用以下命令在Ubuntu上安装这两个库:
```
sudo apt-get install libopencv-dev
sudo apt-get install libdlib-dev
```
2. 加载人脸识别模型
使用Dlib库提供的人脸检测器和68个关键点检测器,需要加载人脸识别模型。可使用以下代码:
```
#include <dlib/opencv.h>
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/image_processing.h>
using namespace dlib;
frontal_face_detector detector = get_frontal_face_detector();
shape_predictor sp;
deserialize("shape_predictor_68_face_landmarks.dat") >> sp;
```
3. 加载人脸数据库
将需要识别的人脸图片保存到人脸数据库中。可使用以下代码加载人脸数据库:
```
std::vector<matrix<rgb_pixel>> faces;
std::vector<std::string> labels;
// Load faces from a directory path
load_image_dataset(faces, labels, "faces");
```
4. 人脸检测和关键点检测
使用Dlib库提供的人脸检测器和68个关键点检测器,对待识别的人脸图像进行处理,提取人脸特征。可使用以下代码:
```
// Load the input image
cv::Mat inputImg = cv::imread("face.jpg");
// Convert the input image to Dlib's format
cv_image<rgb_pixel> dlibImg(inputImg);
// Detect faces in the image
std::vector<rectangle> dets = detector(dlibImg);
// Find the pose of each face
std::vector<full_object_detection> shapes;
for (unsigned long j = 0; j < dets.size(); ++j) {
full_object_detection shape = sp(dlibImg, dets[j]);
shapes.push_back(shape);
}
```
5. 人脸识别
将待识别的人脸特征与人脸数据库中的特征进行比对,找到最相似的人脸。可使用以下代码:
```
// Compute the face descriptor for each face
std::vector<matrix<float,0,1>> faceDescriptors;
for (unsigned long i = 0; i < shapes.size(); ++i) {
matrix<rgb_pixel> faceChip;
extract_image_chip(dlibImg, get_face_chip_details(shapes[i],150,0.25), faceChip);
faceDescriptors.push_back(net(faceChip));
}
// Find the closest match in the database
std::vector<double> distances;
std::string bestLabel;
double bestDistance = 1.0;
for (unsigned long i = 0; i < faces.size(); ++i) {
double distance = length(faceDescriptors[0] - faceDescriptors[i]);
if (distance < bestDistance) {
bestDistance = distance;
bestLabel = labels[i];
}
}
```
以上是使用C++实现人脸识别的基本步骤。可以根据实际需求对代码进行修改和优化。
dlib linux
Dlib is a popular open-source library for machine learning and computer vision tasks, particularly focused on real-time applications. It was initially developed by David Eberly for use in facial recognition and has since expanded to include tools for image processing, optimization, and more. Dlib is primarily designed for C++ but also offers Python bindings, making it accessible to a wider audience.
In Linux, Dlib can be installed using package managers like apt (Ubuntu/Debian) or yum (RHEL/CentOS), by searching for the "dlib" or "dlib-devel" package. Alternatively, you can download the source code from the official GitHub repository (<https://github.com/davisking/dlib>) and build it manually, following the provided instructions.
Some key features of Dlib in Linux include:
1. Face detection and recognition: Dlib is well-known for its robust face detection algorithms, which can be used for tasks like real-time face tracking or authentication.
2. Object detection and localization: The library supports various object detection methods, useful for computer vision applications.
3. Machine learning models: Dlib provides pre-trained models for tasks such as linear regression, support vector machines, and deep neural networks, including CNNs and CNN-based face recognition.
4. Image processing utilities: It includes functions for image resizing, normalization, and other common operations.
To get started with Dlib in Linux, you would typically:
- Install the library: `sudo apt-get install dlib` (for apt-based systems) or `sudo yum install dlib-devel` (for yum-based systems).
- Include the necessary headers and link against the library in your projects.
- Explore the extensive documentation (<http://dlib.net/docs.html>), tutorials, and examples for integration.
阅读全文
相关推荐


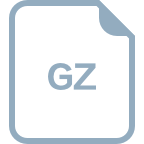


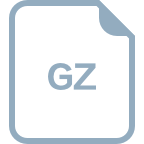

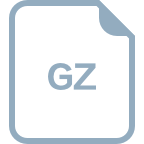







