C++二维装箱算法,箱子可以旋转,返回每个箱子的位置和是否旋转,注意数据是double,每个盒子有ID
时间: 2023-12-18 22:02:05 浏览: 25
二维装箱问题是一个经典的组合优化问题,它可以被描述为:有一些矩形需要放入一些大矩形中,如何将它们放置使得物品利用率最高(即占用空间最小)。
而对于本题,箱子可以旋转,因此需要考虑两种情况:箱子不旋转和箱子旋转90度。
一种常见的解决方案是使用贪心算法。具体步骤如下:
1. 将所有箱子按照面积从大到小排序
2. 从大到小遍历每个箱子,依次将其放入最合适的位置
3. 对于每个箱子,先尝试不旋转,如果放不下再尝试旋转。如果还是放不下,则将其放入新的一行或一列
4. 重复以上步骤直到所有箱子都被放置
对于每个箱子,我们需要记录以下信息:位置(左上角坐标)、是否旋转、箱子ID。
以下是一个可能的实现:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
#include <cmath>
using namespace std;
struct Box {
int id;
double w;
double h;
double area;
bool rotate;
double x;
double y;
Box(int _id, double _w, double _h): id(_id), w(_w), h(_h) {
area = w * h;
rotate = false;
x = 0;
y = 0;
}
};
bool compare(const Box& a, const Box& b) {
return a.area > b.area;
}
void pack(vector<Box>& boxes, double container_w, double container_h) {
double x = 0;
double y = 0;
double row_h = 0;
double col_w = 0;
for (auto& box : boxes) {
if (!box.rotate && box.w <= container_w - col_w) {
// 不旋转,放在当前行
box.x = x + col_w;
box.y = y + row_h;
col_w += box.w;
row_h = max(row_h, box.h);
} else if (box.h <= container_h - row_h) {
// 旋转,放在当前列
box.rotate = true;
box.x = x + col_w;
box.y = y + row_h;
col_w = box.w;
row_h += box.h;
} else if (!box.rotate && box.h <= container_w - col_w) {
// 不旋转,放在新的一列
box.x = x + col_w;
box.y = y;
col_w += box.w;
row_h = box.h;
} else if (box.w <= container_h - row_h) {
// 旋转,放在新的一行
box.rotate = true;
box.x = x;
box.y = y + row_h;
col_w = box.w;
row_h += box.h;
} else {
// 放不下,新起一行或一列
if (container_w - col_w < container_h - row_h) {
// 新起一列
x += col_w;
col_w = box.w;
row_h = box.h;
box.x = x;
box.y = y;
} else {
// 新起一行
y += row_h;
row_h = box.h;
col_w = box.w;
box.x = x;
box.y = y;
}
}
}
}
int main() {
vector<Box> boxes{ Box(1, 4.2, 3.1), Box(2, 2.7, 5.8), Box(3, 3.1, 4.2), Box(4, 5.8, 2.7) };
double container_w = 10;
double container_h = 10;
sort(boxes.begin(), boxes.end(), compare);
pack(boxes, container_w, container_h);
for (auto& box : boxes) {
cout << "Box " << box.id << ":\n"
<< "\tWidth: " << box.w << ", Height: " << box.h << "\n"
<< "\tPosition: (" << box.x << ", " << box.y << ")\n"
<< "\tRotate: " << (box.rotate ? "true" : "false") << endl;
}
return 0;
}
```
以上代码使用了C++11中的auto关键字、lambda表达式等特性,如果不熟悉可以自行查阅相关文档。
相关推荐
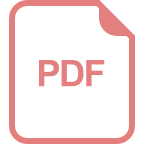
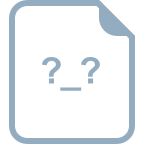














