vue写一个滑动选择器
时间: 2024-05-04 08:15:17 浏览: 155
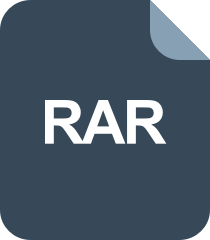
vue.js版双向滑动区间选择器

以下是一个简单的滑动选择器示例,使用Vue进行编写。该滑动选择器适用于选择数字或日期等连续的值。
HTML代码:
```
<template>
<div class="slider-container">
<div class="slider-track" ref="sliderTrack">
<div v-for="value in values" :key="value" class="slider-value" :style="{ left: getOffset(value) + '%' }">{{ value }}</div>
<div class="slider-thumb" :style="{ left: getOffset(selectedValue) + '%' }" ref="sliderThumb"></div>
</div>
</div>
</template>
```
CSS样式:
```
.slider-container {
position: relative;
width: 100%;
height: 50px;
overflow: hidden;
}
.slider-track {
position: absolute;
top: 50%;
left: 0;
right: 0;
transform: translateY(-50%);
height: 2px;
background-color: #ccc;
}
.slider-value {
position: absolute;
top: 50%;
transform: translateY(-50%);
font-size: 12px;
color: #999;
}
.slider-thumb {
position: absolute;
top: 50%;
transform: translateY(-50%);
width: 24px;
height: 24px;
border-radius: 50%;
background-color: #007aff;
box-shadow: 0 1px 3px rgba(0, 0, 0, 0.3);
cursor: pointer;
}
```
JavaScript代码:
```
<script>
export default {
data() {
return {
values: [], // 范围内的值
selectedValue: 0, // 当前选择的值
dragging: false, // 是否正在拖动滑块
startX: 0, // 滑块开始拖动时的X坐标
thumbOffset: 0 // 滑块的偏移量
};
},
mounted() {
// 初始化值
for (let i = 0; i <= 100; i++) {
this.values.push(i);
}
// 监听窗口大小变化,重新计算滑块的偏移量
window.addEventListener('resize', this.handleResize);
// 初始化滑块的偏移量
this.thumbOffset = this.$refs.sliderThumb.offsetWidth / 2;
// 监听滑块的拖动事件
this.$refs.sliderThumb.addEventListener('mousedown', this.handleMouseDown);
document.addEventListener('mousemove', this.handleMouseMove);
document.addEventListener('mouseup', this.handleMouseUp);
},
methods: {
// 获取值在滑块轨道上的偏移量
getOffset(value) {
const range = this.values[this.values.length - 1] - this.values[0];
return ((value - this.values[0]) / range) * 100;
},
// 获取滑块当前所在的值
getValue(offset) {
const range = this.values[this.values.length - 1] - this.values[0];
return Math.round((offset / 100) * range + this.values[0]);
},
// 处理窗口大小变化事件
handleResize() {
this.thumbOffset = this.$refs.sliderThumb.offsetWidth / 2;
},
// 处理滑块的拖动事件
handleMouseDown(event) {
this.dragging = true;
this.startX = event.clientX;
},
handleMouseMove(event) {
if (this.dragging) {
const sliderTrackRect = this.$refs.sliderTrack.getBoundingClientRect();
const thumbLeft = this.$refs.sliderThumb.offsetLeft - sliderTrackRect.left;
const thumbRight = thumbLeft + this.$refs.sliderThumb.offsetWidth;
const sliderTrackWidth = sliderTrackRect.width;
const moveX = event.clientX - this.startX;
const newThumbLeft = thumbLeft + moveX;
const newThumbRight = thumbRight + moveX;
if (newThumbLeft >= 0 && newThumbRight <= sliderTrackWidth) {
this.$refs.sliderThumb.style.left = newThumbLeft + 'px';
this.selectedValue = this.getValue((newThumbLeft + this.thumbOffset) / sliderTrackWidth * 100);
}
this.startX = event.clientX;
}
},
handleMouseUp() {
this.dragging = false;
}
}
};
</script>
```
阅读全文
相关推荐
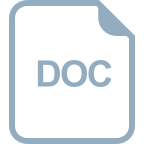
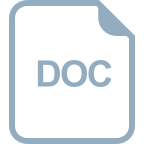
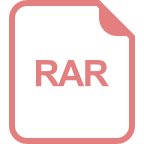





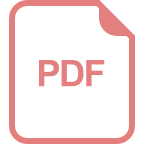
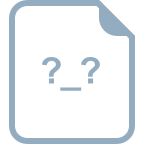
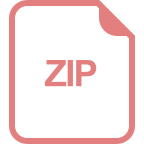



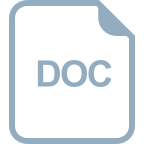
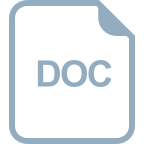