vue 自定义组件 v-model双向绑定、 父子组件同步通信的多种写法
时间: 2023-04-01 14:03:53 浏览: 115
可以使用 prop 和 $emit 实现父子组件之间的通信,也可以使用 provide 和 inject 实现祖先组件向后代组件的通信。另外,还可以使用 Vuex 状态管理库来实现组件之间的状态共享。对于 v-model 的双向绑定,可以通过在子组件中使用 $emit 触发父组件的更新事件,从而实现双向绑定的效果。
相关问题
vue父子组件数据绑定
### Vue 父子组件的数据绑定方法
在 Vue 中,父子组件间的数据交互遵循单向数据流原则。父组件可以通过 `props` 将数据传递给子组件,而子组件则通过 `$emit` 发送事件通知父组件更新状态。
#### 使用 Props 和 Emit 实现双向绑定
当父组件需要监听来自子组件的状态变化时,通常会采用这种模式:
```html
<!-- ParentComponent.vue -->
<template>
<div>
<ChildComponent :message="parentMessage" @update:message="handleUpdate"></ChildComponent>
{{ parentMessage }}
</div>
</template>
<script>
export default {
data() {
return {
parentMessage: 'Hello from parent'
}
},
methods: {
handleUpdate(newVal) {
this.parentMessage = newVal;
}
}
}
</script>
```
```html
<!-- ChildComponent.vue -->
<template>
<input type="text" :value="message" @input="$emit('update:message', $event.target.value)">
</template>
<script>
export default {
props: ['message']
}
</script>
```
这种方法确保了即使是在复杂的嵌套结构下也能保持清晰的数据流向[^1]。
#### 利用 `.sync` 修饰符简化写法
对于某些场景,如果希望更简洁地表达同步关系,则可以考虑使用`.sync`修饰符:
```html
<!-- ParentComponent.vue -->
<template>
<div>
<ChildComponent :message.sync="parentMessage"/>
{{ parentMessage }}
</div>
</template>
```
此时,在子组件内部只需正常触发 update:eventName 即可完成通信过程[^3].
#### 推荐做法:借助 v-model 完成双向绑定
最常用也最为直观的方式就是利用内置的 `v-model` 指令来进行双向绑定操作,这实际上是上述两种方案的一种语法糖形式:
```html
<!-- ParentComponent.vue -->
<template>
<div>
<ChildComponent v-model="parentMessage"/>
{{ parentMessage }}
</div>
</template>
```
而在子组件中只需要定义好相应的 input event 来响应输入行为即可:
```html
<!-- ChildComponent.vue -->
<template>
<input type="text" :value="modelValue" @input="$emit('update:modelValue', $event.target.value)" />
</template>
<script>
export default {
props: ['modelValue'],
};
</script>
```
这种方式不仅提高了代码的可读性和维护性,同时也减少了模板中的冗余逻辑.
.sync v-model
.sync修饰符与v-model在Vue自定义组件中的区别是什么?
.sync修饰符是用于实现父组件向子组件传递数据并实时更新的功能。在正常的父传子情况下,我们可以使用v-bind将数据传递给子组件,但是在子组件内部如果要修改这个数据,就需要通过$emit方法向父组件发送事件并传递新的值。而使用.sync修饰符之后,我们可以直接在子组件中使用v-model来接收父组件传递的数据,并通过触发update事件来实时更新父组件的数据。这样就省去了显式地使用$emit方法来发送事件的步骤。
例如,使用.sync修饰符的父传子情况:
<com1 :a.sync="num"></com1>
等价于
<com1 :a="num" @update:a="val=>num=val"></com1>
其中,num是父组件中的数据,通过:a.sync将num传递给子组件的a属性,并且监听update:a事件,当子组件内部通过v-model修改了a的值时,会自动触发update:a事件,将新的值传递回父组件并实时更新num的值。
而v-model是Vue提供的一种语法糖,用于实现双向数据绑定。通常情况下,我们在子组件中使用v-model来接收父组件传递的数据,并通过触发input事件来将新的值传递回父组件。使用v-model的原理是侦听value属性和input事件。
例如,使用v-model的情况:
<son v-model="num"></son>
等价于
<son :value="num" @input="val=>this.num=val"></son>
其中,num是父组件中的数据,通过:value将num传递给子组件的value属性,并且监听input事件,当子组件内部通过$emit方法触发input事件时,将新的值传递回父组件并实时更新num的值。
综上所述,.sync修饰符与v-model在实现父子组件之间数据的双向绑定上有所区别。.sync修饰符可以省去显式地使用$emit方法发送事件的步骤,而v-model是Vue提供的一种语法糖,用于简化双向数据绑定的写法。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [详解VUE自定义组件中用.sync修饰符与v-model的区别](https://download.csdn.net/download/weixin_38629391/12758140)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *2* [.sync与v-model的区别](https://blog.csdn.net/weixin_48837605/article/details/119616396)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *3* [VUE中 .sync 修饰符 和 v-model区别](https://blog.csdn.net/weixin_59229847/article/details/120815524)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
[ .reference_list ]
阅读全文
相关推荐
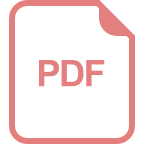
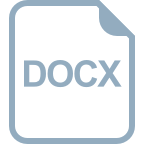
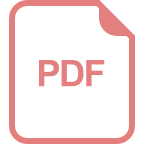
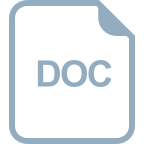

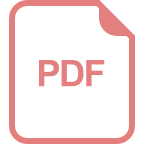
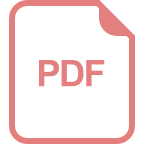
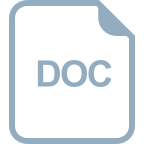
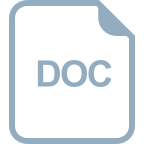
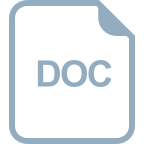
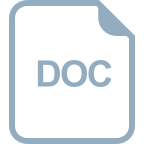
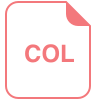
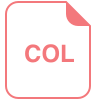
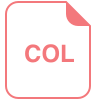

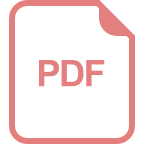
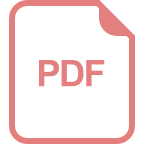