帮我用 Python写一个爬取网站小说的爬虫程序
时间: 2023-05-30 15:07:27 浏览: 296
以下是一个简单的Python爬虫程序,用于爬取小说网站上的小说。
```
import requests
from bs4 import BeautifulSoup
# 设置需要爬取的小说网站URL
url = 'https://www.example.com/novel'
# 发送请求并获取响应
response = requests.get(url)
# 解析HTML响应内容
soup = BeautifulSoup(response.text, 'html.parser')
# 找到小说内容所在的标签
novel_content = soup.find('div', class_='novel-content')
# 打印小说内容
print(novel_content.text)
```
以上代码只是一个简单的示例,实际上爬取网站小说需要考虑到很多方面,比如反爬虫机制、网站结构的变化等等。因此,建议使用更加完善的爬虫框架,如Scrapy等。同时也需要注意法律法规,不得在未经授权的情况下获取版权作品。
相关问题
帮我写一个可以实现关键词爬取的Python爬虫程序
要编写一个简单的Python爬虫程序来抓取网页上的关键词,我们可以使用如BeautifulSoup库配合requests库来解析HTML内容。以下是一个基本的示例,假设我们要从一个网页抓取标题(这通常包含关键词):
```python
import requests
from bs4 import BeautifulSoup
def keyword_scraper(url, target_keyword):
# 发送HTTP请求获取网页内容
response = requests.get(url)
# 检查请求是否成功
if response.status_code == 200:
# 使用BeautifulSoup解析HTML
soup = BeautifulSoup(response.text, 'html.parser')
# 查找包含目标关键词的元素(例如,假设标题标签通常是<h1>或<h2>)
titles = soup.find_all(['h1', 'h2'], text=lambda t: target_keyword in t.get_text())
# 打印或保存找到的结果
for title in titles:
print(f"Title containing '{target_keyword}': {title.get_text()}")
else:
print(f"Failed to fetch URL. Status code: {response.status_code}")
# 示例使用
keyword_scraper('https://example.com', '关键词')
用python写一个爬取漫画网站的程序
编写一个Python爬虫程序来抓取漫画网站的内容,特别是对于受到保护的付费内容,我们需要谨慎并遵循网站的robots.txt规则。以下是一个基本框架示例,假设我们有一个简单的网站没有采用反爬虫技术:
```python
import requests
from bs4 import BeautifulSoup
# 漫画网站URL
comic_url = 'https://example.com/manga/'
def get_content(url):
# 发送GET请求
response = requests.get(url)
# 判断请求状态码,若非200则返回错误信息
if response.status_code != 200:
return "Error: Unable to access the page."
# 使用BeautifulSoup解析HTML内容
soup = BeautifulSoup(response.text, 'html.parser')
# 找到包含漫画图片的标签,这里仅作示例,实际可能更复杂
image_tags = soup.find_all('img', class_='comic-image') # 假设图片在class为'comic-image'的元素里
for img in image_tags:
img_url = img['src'] # 获取图片链接
download_image(img_url) # 下载图片函数,这里略去
def download_image(img_url):
with open('downloaded_images/' + img_url.split('/')[-1], 'wb') as f:
f.write(requests.get(img_url).content)
# 开始爬取
get_content(comic_url)
```
请注意,这只是一个非常基础的例子,实际操作中可能需要处理更多情况,如登录验证、动态加载内容、反爬虫策略、数据持久化等。而且,一定要确保你的行为符合法律和网站的规定。
阅读全文
相关推荐
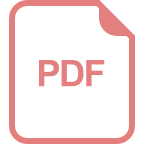
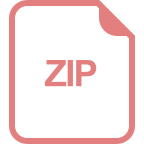
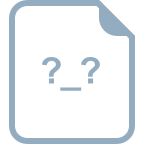













