用python写一个爬取csdn首页的爬虫程序
时间: 2023-12-04 11:02:33 浏览: 105
好的,以下是一个简单的Python爬虫程序,可以爬取CSDN首页的文章标题和链接:
```python
import requests
from bs4 import BeautifulSoup
url = "https://www.csdn.net/"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
articles = soup.find_all("div", class_="title")
for article in articles:
title = article.find("a").string.strip()
link = article.find("a")["href"]
print(title)
print(link)
```
这个程序使用了`requests`库和`BeautifulSoup`库,首先通过`requests.get()`方法获取CSDN首页的HTML内容,然后使用`BeautifulSoup`解析HTML内容,找到所有标题的`div`标签,遍历每个标签,提取出标题和链接,最后输出到控制台。
相关问题
用python写一个爬虫,爬取csdn
的文章标题和链接
```python
import requests
from bs4 import BeautifulSoup
url = 'https://blog.csdn.net/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for article in soup.select('.article-list .title h2 a'):
print(article.text)
print(article['href'])
```
解释:
1. 首先导入`requests`和`BeautifulSoup`模块。
2. 定义要爬取的网址。
3. 发送请求并获取响应。
4. 使用`BeautifulSoup`解析响应文本。
5. 遍历文章列表中的每一篇文章,并输出标题和链接。其中,`soup.select('.article-list .title h2 a')`表示选择所有class为`article-list`和`title`的元素下的`h2`标签下的`a`标签。
使用python写一个csdn的爬虫程序
以下是一个简单的爬取CSDN博客的程序示例:
```python
import requests
from bs4 import BeautifulSoup
# 设置请求头,模拟浏览器访问
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 设置要爬取的博客地址
url = "https://blog.csdn.net/xxxxxx/article/details/xxxxxx"
# 发送请求,获取响应内容
res = requests.get(url, headers=headers)
res.encoding = 'utf-8'
html = res.text
# 解析HTML内容
soup = BeautifulSoup(html, 'html.parser')
title = soup.find('title').text
content = soup.find('div', class_='markdown_views').text
# 输出结果
print("标题:", title)
print("内容:", content)
```
以上代码中,使用了requests和BeautifulSoup库来发送请求、解析HTML内容。需要注意的是,需要设置请求头来模拟浏览器访问,否则可能会被CSDN禁止访问。根据需要爬取的内容不同,可以修改代码中的选择器来获取对应的信息。
阅读全文
相关推荐
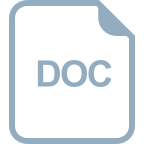
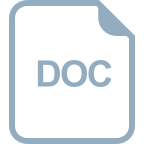
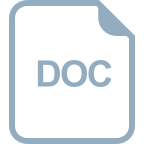
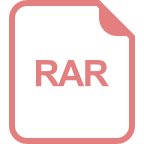

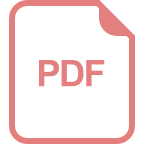
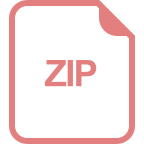








