如何在PCF8591芯片上编写AD转换函数
时间: 2023-05-22 11:06:06 浏览: 138
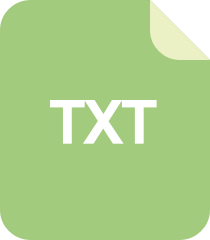
PCF8591芯片的AD转换
要在PCF8591芯片上编写AD转换函数,您需要使用I2C接口与芯片进行通信。首先,您需要在您的代码中包含I2C库,并初始化I2C接口。然后,您可以使用PCF8591的地址来初始化芯片并设置转换通道。最后,您可以使用I2C接口从芯片读取数据并将其转换为AD值。以下是用C语言编写的示例代码:
#include <stdio.h>
#include <errno.h>
#include <stdlib.h>
#include <stdint.h>
#include <stdbool.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/ioctl.h>
#include <linux/i2c-dev.h>
// Define the I2C address of the PCF8591 chip
#define PCF8591_ADDRESS 0x48
// Define the registers of the PCF8591 chip
#define PCF8591_CTRL_REG 0x40
#define PCF8591_ADC_REG 0x00
int main() {
int i2c_fd;
uint8_t buffer[2];
// Open the I2C interface
i2c_fd = open("/dev/i2c-1", O_RDWR);
if (i2c_fd < 0) {
perror("Failed to open I2C interface");
exit(EXIT_FAILURE);
}
// Set the I2C address of the PCF8591 chip
if (ioctl(i2c_fd, I2C_SLAVE, PCF8591_ADDRESS) < 0) {
perror("Failed to set I2C address");
exit(EXIT_FAILURE);
}
// Configure the PCF8591 chip for analog input on channel 0
buffer[0] = PCF8591_CTRL_REG;
buffer[1] = 0x00; // Set to read from channel 0
// Write the configuration to the PCF8591 chip
if (write(i2c_fd, buffer, 2) != 2) {
perror("Failed to write to PCF8591 chip");
exit(EXIT_FAILURE);
}
// Read the ADC value from the PCF8591 chip
buffer[0] = PCF8591_ADC_REG;
if (write(i2c_fd, buffer, 1) != 1) {
perror("Failed to write to PCF8591 chip");
exit(EXIT_FAILURE);
}
if (read(i2c_fd, buffer, 1) != 1) {
perror("Failed to read from PCF8591 chip");
exit(EXIT_FAILURE);
}
// Convert the ADC value to a voltage
float voltage = ((float)buffer[0] / 255.0) * 5.0;
// Print the ADC value and voltage to the console
printf("ADC value: %d\n", buffer[0]);
printf("Voltage: %.3fV\n", voltage);
// Close the I2C interface
close(i2c_fd);
return 0;
}
阅读全文
相关推荐
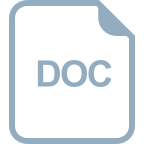
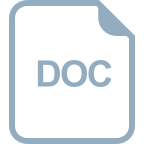
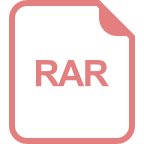
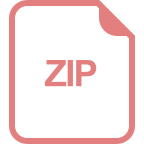
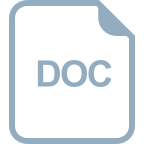
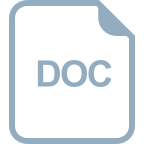
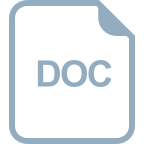
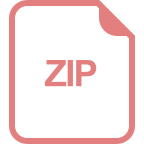
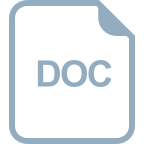
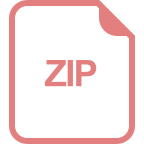
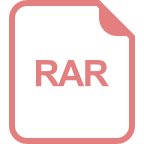
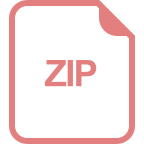
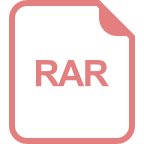
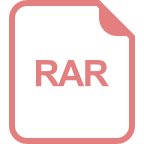
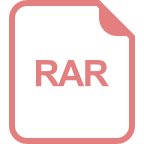
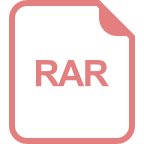
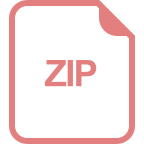
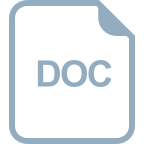