并行遗传算法的python实现
时间: 2023-09-10 11:06:20 浏览: 106
并行遗传算法(Parallel Genetic Algorithm,PGA)是一种应用并行计算技术进行优化的遗传算法。在PGA中,多个遗传算法在同一时刻并行地运行,通过交换信息和协作搜索,提高了全局搜索能力,从而提高了算法的收敛速度和精度。
下面是一个简单的Python实现:
```python
import numpy as np
import multiprocessing as mp
import time
# 目标函数
def objective_function(x):
return np.sum(np.square(x))
# 个体类
class Individual:
def __init__(self, x):
self.x = x
self.fitness = objective_function(x)
# 交叉操作
def crossover(self, other):
alpha = np.random.rand(len(self.x))
child_x = alpha * self.x + (1 - alpha) * other.x
return Individual(child_x)
# 变异操作
def mutation(self):
sigma = 0.1
child_x = self.x + sigma * np.random.randn(len(self.x))
return Individual(child_x)
# 遗传算法类
class GeneticAlgorithm:
def __init__(self, pop_size, num_generations, num_parents, num_children):
self.pop_size = pop_size
self.num_generations = num_generations
self.num_parents = num_parents
self.num_children = num_children
# 初始化种群
def initialize_population(self, num_variables):
population = []
for i in range(self.pop_size):
x = np.random.randn(num_variables)
individual = Individual(x)
population.append(individual)
return population
# 选择操作
def selection(self, population):
fitnesses = np.array([individual.fitness for individual in population])
fitnesses = 1 / (1 + fitnesses)
fitnesses /= np.sum(fitnesses)
parents_idx = np.random.choice(np.arange(self.pop_size), size=self.num_parents, replace=False, p=fitnesses)
return [population[idx] for idx in parents_idx]
# 进化操作
def evolution(self, parents):
children = []
while len(children) < self.num_children:
parent1, parent2 = np.random.choice(parents, size=2, replace=False)
child = parent1.crossover(parent2).mutation()
children.append(child)
return children
# 并行运行遗传算法
def run_parallel(self, num_processes):
# 初始化种群
population = self.initialize_population(num_variables=2)
for generation in range(self.num_generations):
# 选择父代
parents = self.selection(population)
# 并行进化子代
pool = mp.Pool(num_processes)
results = [pool.apply_async(self.evolution, args=(parents,)) for _ in range(num_processes)]
children = []
for result in results:
children += result.get()
pool.close()
# 合并父代和子代
population = parents + children
# 评估种群中每个个体的适应度
fitnesses = np.array([individual.fitness for individual in population])
# 打印当前最优解
best_idx = np.argmin(fitnesses)
print(f"Generation {generation}, Best Fitness: {fitnesses[best_idx]}, Best Solution: {population[best_idx].x}")
if __name__ == '__main__':
start_time = time.time()
ga = GeneticAlgorithm(pop_size=100, num_generations=50, num_parents=50, num_children=50)
ga.run_parallel(num_processes=4)
end_time = time.time()
print(f"Total Time: {end_time - start_time}")
```
在上面的代码中,我们首先定义了一个目标函数`objective_function`,接着定义了一个个体类`Individual`,其中包括交叉和变异操作。然后,我们定义了一个遗传算法类`GeneticAlgorithm`,其中包括初始化种群、选择、进化等操作。最后,我们使用`multiprocessing`模块实现并行运行遗传算法,其中`num_processes`参数指定了并行进化的进程数。
在运行程序时,我们可以看到每一代中最优解的变化情况,并且可以观察到并行运行遗传算法的速度明显快于串行运行。
需要注意的是,PGA的并行效果取决于硬件环境和问题特征。在某些情况下,并行运行可能会降低算法的性能而不是提高性能。因此,我们需要对具体问题进行分析和实验,确定最优的并行策略。
阅读全文
相关推荐
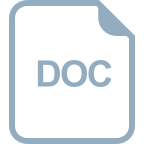
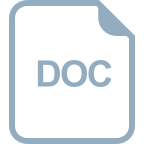
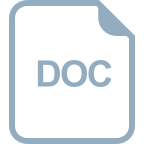
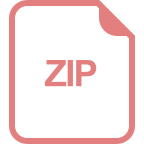
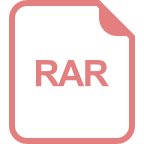
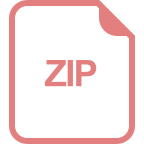
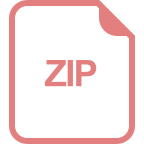
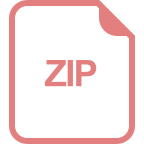
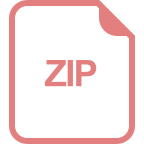
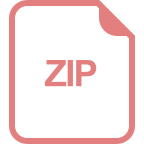
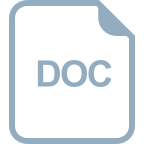







