springboot使用@Value引入配置文件的数据;但是在有的类中引入的时候为null;其他类就是正常的
时间: 2024-05-23 10:11:13 浏览: 11
如果在某些类中使用 `@Value` 注解引入配置文件数据时为 null,但其他类中正常工作,则可能是以下原因之一:
1. 未在该类上添加 `@Component` 或 `@Service` 注解:在将 `@Value` 注解用于类成员变量时,该类必须是 Spring 容器的一个组件,即必须使用 `@Component` 或 `@Service` 注解标识该类。如果未添加这些注解,则 Spring 容器不会对该类进行实例化,导致无法注入配置文件数据。因此,请确保在使用 `@Value` 注解的类上添加了适当的注解。
2. 配置文件中的属性名拼写错误:如果您在配置文件中拼写了属性名,则在使用 `@Value` 注解引用该属性时,Spring 将无法找到它,并将其设置为 null。因此,请确保属性名正确拼写。
3. 配置文件中的属性值为空:如果您在配置文件中没有设置属性的值,则在使用 `@Value` 注解引用该属性时,Spring 将该属性设置为 null。因此,请确保在配置文件中设置了属性值。
4. 该类未被正确扫描:如果您在配置文件中使用了自定义的包扫描路径,则必须确保该类被正确扫描。否则,Spring 容器将无法实例化该类,并且 `@Value` 注解将无法注入属性值。因此,请确保您的自定义扫描路径正确,并且包含使用 `@Value` 注解的类。
希望这些解决方案可以帮助您解决问题。
相关问题
静态类中如何读取springboot yaml文件配置信息
静态类中可以通过使用Spring提供的静态方法读取Spring Boot的YAML配置文件信息。具体实现方式如下:
1. 在静态类中引入静态方法:org.springframework.boot.SpringApplication
2. 通过SpringApplication类的静态方法load方法读取指定 YAML 配置文件中的配置属性。具体代码如下:
```
import org.springframework.boot.SpringApplication;
import org.springframework.boot.env.YamlPropertySourceLoader;
import org.springframework.core.env.PropertySource;
import org.springframework.core.io.ClassPathResource;
import java.io.IOException;
public class YamlUtils {
private static PropertySource<?> getPropertySource(String name) throws IOException {
ClassPathResource resource = new ClassPathResource(name);
YamlPropertySourceLoader loader = new YamlPropertySourceLoader();
PropertySource<?> propertySource = loader.load(name, resource).get(0);
return propertySource;
}
public static String getString(String name){
try {
return (String) getPropertySource("application.yml").getProperty(name);
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
public static int getInt(String name) {
try {
return Integer.valueOf(getString(name));
} catch (Exception e) {
e.printStackTrace();
return 0;
}
}
}
```
3. 调用YamlUtils类的方法即可读取配置信息。
注意:在静态类中读取配置信息时,需要使用Spring相关的组件,如SpringApplication等,所以需要引入相关的Spring依赖。
springboot使用引入的ssl包,报错找不到bean
这个问题可能是由于您没有正确配置SSL相关的bean导致的。您可以尝试检查一下以下几个方面:
1. 检查您的SSL证书文件是否正确,并且已经被正确地加载。您可以使用以下代码片段加载证书文件:
```java
@Configuration
public class SSLConfig {
@Value("${server.ssl.key-store}")
private Resource keyStore;
@Value("${server.ssl.key-store-password}")
private String keyStorePassword;
@Value("${server.ssl.trust-store}")
private Resource trustStore;
@Value("${server.ssl.trust-store-password}")
private String trustStorePassword;
@Bean
public SSLContext sslContext() throws Exception {
KeyStore keyStore = KeyStore.getInstance(KeyStore.getDefaultType());
keyStore.load(this.keyStore.getInputStream(), keyStorePassword.toCharArray());
KeyStore trustStore = KeyStore.getInstance(KeyStore.getDefaultType());
trustStore.load(this.trustStore.getInputStream(), trustStorePassword.toCharArray());
return SSLContexts.custom()
.loadKeyMaterial(keyStore, keyStorePassword.toCharArray())
.loadTrustMaterial(trustStore, null)
.build();
}
}
```
2. 检查您的SSL相关的bean是否正确地被注入到了Spring Boot的容器中。您可以在您的配置类中使用注解 @ImportResource 导入您的 XML 配置文件,或者使用注解 @ComponentScan 扫描您的SSL相关的类。
如果以上两个方面都没有问题,您可以尝试查看一下错误日志,看看具体是哪个bean没有找到,并且排查一下原因。如果您还有其他问题,欢迎继续提问。
相关推荐
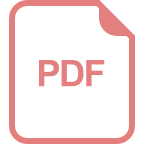
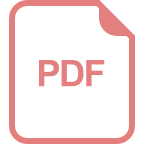
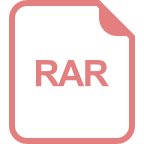












