pytorch将低纬度向量融入高纬度向量中
时间: 2023-12-19 16:52:47 浏览: 32
PyTorch 提供了多种方式将低维度向量融合到高维度向量中,以下是其中的一些方法:
1. 使用 torch.unsqueeze() 函数将低维度向量扩展为高维度向量中的一个维度,然后使用 torch.cat() 函数将两个向量拼接在一起。
```python
import torch
low_dim = torch.tensor([1, 2, 3])
high_dim = torch.rand((5, 4))
# 将 low_dim 扩展为 (3, 1) 的张量
low_dim = low_dim.unsqueeze(1)
# 拼接 high_dim 和 low_dim 的最后一个维度
result = torch.cat((high_dim, low_dim), dim=1)
```
2. 使用 torch.stack() 函数将两个向量堆叠在一起,创建一个新的维度。
```python
import torch
low_dim = torch.tensor([1, 2, 3])
high_dim = torch.rand((5, 4))
# 将两个张量沿着新的维度堆叠
result = torch.stack((high_dim, low_dim), dim=2)
```
3. 使用 torch.repeat() 函数将低维度向量复制为高维度向量的一部分,然后使用 torch.cat() 函数将两个向量拼接在一起。
```python
import torch
low_dim = torch.tensor([1, 2, 3])
high_dim = torch.rand((5, 4))
# 将 low_dim 复制 5 次,变为 (5, 3) 的张量
low_dim = low_dim.repeat(5, 1)
# 拼接 high_dim 和 low_dim 的最后一个维度
result = torch.cat((high_dim, low_dim), dim=1)
```
以上三种方法都可以将低维度向量融合到高维度向量中,选择哪种方法取决于具体需求和数据结构。
相关推荐
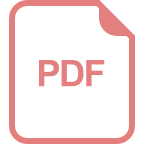














