function test(name){ this.name=name; return "你好," +this.name +'<br/>'; } var rval = test('Tom'); document.writeln( rval ); var obj = new test('Mary'); for(var i in obj){ document.writeln(i+":"+obj[i]); }
时间: 2024-01-18 15:01:57 浏览: 30
这段代码的输出结果为:
你好,Tom
name:Mary
首先,调用test函数并传入参数'Tom',返回字符串"你好,Tom",并将其赋值给变量rval,输出该字符串。
然后,使用new关键字创建test函数的实例对象obj,并传入参数'Mary'。此时,test函数内部的this指向创建的实例对象obj,将参数name赋值给实例对象的属性name。
最后,使用for...in循环遍历实例对象obj的属性,输出name属性及其值"Mary"。注意,函数内部的return语句只会影响函数的返回值,并不会影响函数内部this指向的对象。
相关问题
const int &rval=rval1;
这段代码是将rval引用初始化为rval1的常量引用。这意味着rval将永远引用rval1,并且不能通过rval更改rval1的值。这是因为rval是一个常量引用,它只能读取rval1的值,而不能修改它。以下是一个示例代码:
```cpp
#include <iostream>
using namespace std;
int main() {
int i = 42;
const int &rval = i;
cout << "rval = " << rval << endl; // 输出:rval = 42
i = 0;
cout << "rval = " << rval << endl; // 输出:rval = 0
return 0;
}
```
def turnvalue(Value): if(Value.ctype == 1): #string _str = Value.value _str.lstrip(' '); _str.rstrip(' '); if('0x' in _str): tmp = _str.split('0x') #print 'tmp : ',tmp rval = tmp[1]; #print 'tmp[0] ',tmp[0] #print 'rval : ',rval if(tmp[0] == ''): rval = "%s%s"%("'h",rval) else: rval = _str; else: rval = _str elif(Value.ctype == 2): #number _num = Value.value rval = int(_num) if(_num != rval): #real type #print '_num is ',_num rval = _num else: #int type rval = str(rval) elif(Value.ctype == 0): #empty rval = ' ' else: #other type print(inRed("[Error] value.ctype is not right")) sys.exit() return rval
这是一个Python的函数定义,函数名为turnvalue,参数为Value。函数内部使用了Python的if条件语句,判断Value的ctype是否为1。如果是1,函数会执行一些操作(这里没有给出);否则函数不做任何操作。
相关推荐
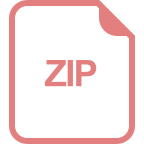
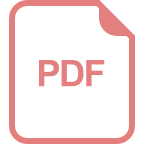












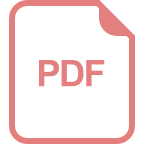