用C++编写,头文件使用iostream
时间: 2023-12-21 08:04:32 浏览: 78
好的,C++ 语言中的链表可以使用 `struct` 或 `class` 来定义,我这里使用 `class` 来实现。代码如下:
```c++
#include <iostream>
using namespace std;
class Student {
public:
int num;
string name;
Student *next;
Student(int num, string name) {
this->num = num;
this->name = name;
this->next = NULL;
}
};
class LinkedList {
public:
Student *head;
LinkedList() {
this->head = NULL;
}
void add(Student *node) {
if (this->head == NULL) {
this->head = node;
} else {
Student *p = this->head;
while (p->next != NULL) {
p = p->next;
}
p->next = node;
}
}
void print() {
Student *p = this->head;
int i = 1;
while (p != NULL) {
cout << "第" << i << "个学生的学号和姓名:" << p->num << " " << p->name << endl;
p = p->next;
i++;
}
}
};
int main() {
LinkedList *list = new LinkedList();
list->add(new Student(1, "张三"));
list->add(new Student(2, "李四"));
list->add(new Student(3, "王五"));
list->print();
return 0;
}
```
这个程序会输出以下结果:
```
第1个学生的学号和姓名:1 张三
第2个学生的学号和姓名:2 李四
第3个学生的学号和姓名:3 王五
```
在这个程序中,我们使用了 `class` 来定义了两个类:`Student` 和 `LinkedList`。 `Student` 类表示链表中的一个结点, `LinkedList` 类表示整个链表。我们在 `main()` 函数中创建了一个 `LinkedList` 类的对象 `list`,然后使用 `list->add()` 方法向链表中添加了三个学生结点,最后使用 `list->print()` 方法输出链表中的所有结点。
阅读全文
相关推荐
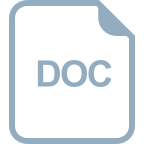
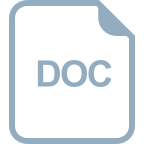
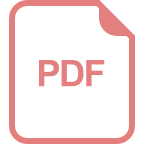
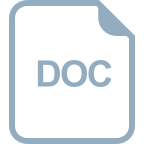
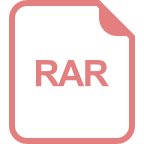
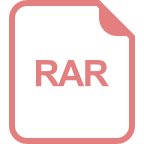
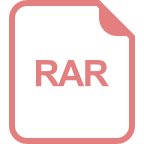
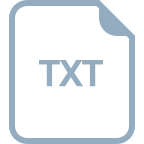
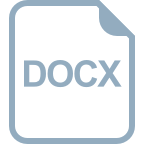
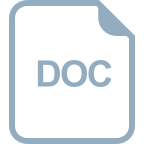
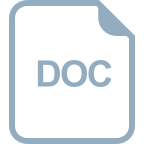
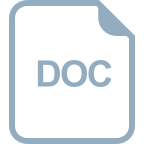


