c#将第一次测试数据(测试总数,ok数,ng数,合格率)数据库,下此开启软件,数据显示到相应的组件中,继续测试,数据继续增加,关闭软件时,测试数据更新为第二次的测试数据,再次开启软件,显示更新后的数据
时间: 2024-05-10 20:16:22 浏览: 184
要实现这个功能,您可以使用以下步骤:
1. 建立一个数据库来存储测试数据,包括测试总数,OK数,NG数和合格率。
2. 在软件启动时,从数据库中读取第一次测试数据,并将其显示在相应的组件中。
3. 在测试过程中,您可以将每个测试的结果(OK或NG)存储在内存中的一个变量中,并在测试完成后更新内存变量中的测试数据。
4. 在软件关闭时,将内存变量中的测试数据更新到数据库中,并将内存变量清空。
5. 下一次启动软件时,从数据库中读取最新的测试数据并将其显示在相应的组件中。
以下是一个示例代码:
```csharp
using System.Data.SqlClient;
namespace TestApp
{
public class TestData
{
public int Total { get; set; }
public int OK { get; set; }
public int NG { get; set; }
public double PassRate { get; set; }
}
public class TestManager
{
private string _connectionString = "Data Source=(local);Initial Catalog=TestDB;Integrated Security=True";
private TestData _testData = new TestData();
public void LoadTestData()
{
using (SqlConnection connection = new SqlConnection(_connectionString))
{
connection.Open();
SqlCommand command = connection.CreateCommand();
command.CommandText = "SELECT Total, OK, NG, PassRate FROM TestResult ORDER BY TestDate DESC";
SqlDataReader reader = command.ExecuteReader();
if (reader.Read())
{
_testData.Total = reader.GetInt32(0);
_testData.OK = reader.GetInt32(1);
_testData.NG = reader.GetInt32(2);
_testData.PassRate = reader.GetDouble(3);
}
reader.Close();
}
}
public void SaveTestData()
{
using (SqlConnection connection = new SqlConnection(_connectionString))
{
connection.Open();
SqlCommand command = connection.CreateCommand();
command.CommandText = "INSERT INTO TestResult (Total, OK, NG, PassRate, TestDate) VALUES (@Total, @OK, @NG, @PassRate, GETDATE())";
command.Parameters.AddWithValue("@Total", _testData.Total);
command.Parameters.AddWithValue("@OK", _testData.OK);
command.Parameters.AddWithValue("@NG", _testData.NG);
command.Parameters.AddWithValue("@PassRate", _testData.PassRate);
command.ExecuteNonQuery();
}
}
public void UpdateTestResult(bool isOK)
{
_testData.Total++;
if (isOK)
{
_testData.OK++;
}
else
{
_testData.NG++;
}
_testData.PassRate = (double)_testData.OK / _testData.Total;
}
public void ClearTestData()
{
_testData = new TestData();
}
public TestData GetTestData()
{
return _testData;
}
}
}
```
在上面的示例代码中,我们创建了一个TestManager类来管理测试数据。在LoadTestData方法中,我们从数据库中读取最新的测试数据,如果有的话。在SaveTestData方法中,我们将当前测试数据保存到数据库中。在UpdateTestResult方法中,我们根据测试结果更新测试数据。在ClearTestData方法中,我们清空内存变量中的测试数据。在GetTestData方法中,我们返回当前测试数据。
阅读全文
相关推荐

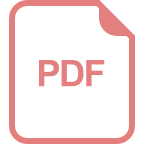
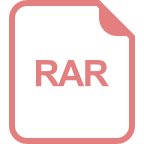
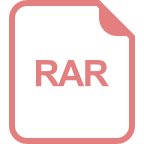
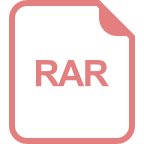
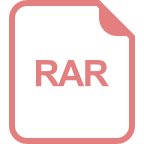
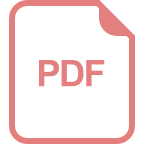
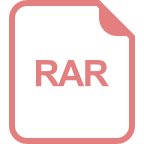
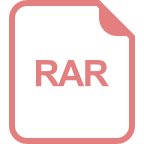
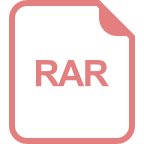
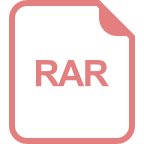
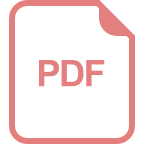
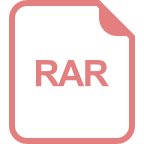
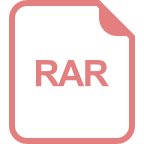
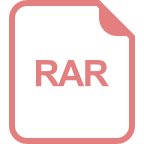