java替换zip中文件
时间: 2024-01-28 22:16:00 浏览: 25
您可以使用Java的ZipInputStream和ZipOutputStream类来实现替换Zip文件中的文件。
具体步骤如下:
1. 创建一个ZipInputStream对象,读取原始的Zip文件。
2. 创建一个ZipOutputStream对象,用于写入修改后的Zip文件。
3. 遍历Zip文件中的每个条目(ZipEntry),如果条目的名称与要替换的文件名称相同,则跳过该条目,否则将该条目写入ZipOutputStream对象中。
4. 创建一个新的ZipEntry对象,将要替换的文件写入ZipOutputStream对象中。
5. 关闭ZipInputStream和ZipOutputStream对象。
以下是一个示例代码,用于替换Zip文件中名为"file.txt"的文件:
```java
import java.io.*;
import java.util.zip.*;
public class ZipReplaceExample {
public static void main(String[] args) throws IOException {
// 原始的Zip文件
File zipFile = new File("example.zip");
// 要替换的文件
File replaceFile = new File("replace.txt");
// 创建ZipInputStream对象,读取原始的Zip文件
ZipInputStream zipIn = new ZipInputStream(new FileInputStream(zipFile));
// 创建ZipOutputStream对象,用于写入修改后的Zip文件
ZipOutputStream zipOut = new ZipOutputStream(new FileOutputStream("example_replaced.zip"));
// 遍历Zip文件中的每个条目
ZipEntry entry;
while ((entry = zipIn.getNextEntry()) != null) {
// 如果条目的名称与要替换的文件名称相同,则跳过该条目
if (entry.getName().equals("file.txt")) {
continue;
}
// 将该条目写入ZipOutputStream对象中
zipOut.putNextEntry(entry);
byte[] buffer = new byte[1024];
int len;
while ((len = zipIn.read(buffer)) > 0) {
zipOut.write(buffer, 0, len);
}
}
// 创建一个新的ZipEntry对象,将要替换的文件写入ZipOutputStream对象中
ZipEntry newEntry = new ZipEntry("file.txt");
zipOut.putNextEntry(newEntry);
FileInputStream replaceIn = new FileInputStream(replaceFile);
byte[] buffer = new byte[1024];
int len;
while ((len = replaceIn.read(buffer)) > 0) {
zipOut.write(buffer, 0, len);
}
replaceIn.close();
// 关闭ZipInputStream和ZipOutputStream对象
zipIn.close();
zipOut.close();
}
}
```
相关推荐
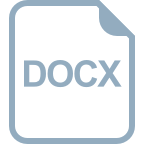












